Skills Required for Python Full Stack Developer
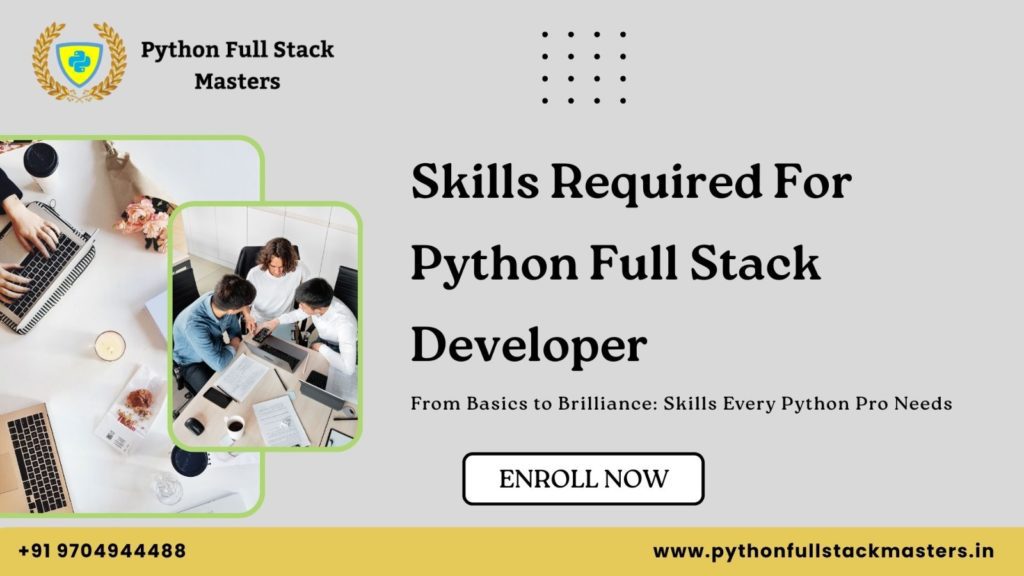
Introduction
Overview of Skills Required for Python Full Stack Development
- Full stack development refers to the ability to work on both the frontend and backend of a web application. A Full Stack Developer is responsible for designing, developing, and maintaining the entire application, ensuring seamless integration between the user interface and the server-side logic.
- Web applications typically consist of two main parts:
- Frontend (Client-Side) – The visual interface that users interact with.
- Backend (Server-Side) – The logic, database, and API layers that process and store data.
- A Python Full Stack Developer specializes in using Python for backend development while working with frontend technologies like HTML, CSS, JavaScript, and various frameworks to build complete applications.
Why Python is Popular for Full Stack Development
- Python has become one of the most widely used programming languages for web development due to its:
- Readability and Simplicity – Python has an easy-to-read syntax that allows developers to write clean and maintainable code.
- Rich Ecosystem of Libraries and Frameworks – Python offers powerful web frameworks like Django and Flask, which simplify backend development.
- Scalability and Performance – Python supports both small and large-scale applications, making it ideal for startups and enterprises alike.
- Cross-Platform Compatibility – Python runs on various platforms like Windows, Linux, and macOS.
- Strong Community Support – Python developers benefit from a large, active community that continuously contributes to its development.
- The Growing Demand for Python Full Stack Developers
- The demand for Python Full Stack Developers is skyrocketing due to:
- The increased adoption of Python-based web frameworks by companies.
- The rise of AI, machine learning, and data-driven applications, where Python plays a key role.
- The need for versatile developers who can work on both frontend and backend.
- According to industry reports, Python Full Stack Developers earn higher-than-average salaries and have numerous career opportunities, ranging from web development and cloud computing to AI and DevOps.
2. Understanding Skills Required for Python Full Stack Developer
Before diving into the required skills, it is essential to understand what full stack development entails.
Definition of Full Stack Development
- Full stack development means handling both the frontend (user-facing) and backend (server-side) of a web application.
- The Frontend-Backend Relationship
- A web application consists of:
- Frontend (Client-Side): Users interact with elements like buttons, forms, and menus.
- Backend (Server-Side): Handles business logic, database operations, and API interactions.
- The frontend (HTML, CSS, JavaScript) displays the form and collects user input.
- The backend (Python, Django, Flask) processes the request, stores data in the database, and sends a response.
Technologies Involved in Full Stack Development
- A Python Full Stack Developer needs to be proficient in multiple technologies, including:
- Frontend: HTML, CSS, JavaScript, React.js, Vue.js
- Backend: Python, Django, Flask, FastAPI
- Database: PostgreSQL, MySQL, MongoDB, SQLite
- APIs: RESTful API, GraphQL
- Version Control: Git, GitHub, GitLab
- Deployment: AWS, Heroku, Docker, Kubernetes
- Full stack development is a multi-disciplinary role that requires continuous learning and adaptation to new technologies.
3. Essential Frontend Skills
A Python Full Stack Developer must have a solid understanding of frontend technologies to create user-friendly and visually appealing web applications.
Why Frontend Skills Matter
- The frontend is the first thing users see and interact with. A poorly designed UI/UX can lead to high bounce rates and low user engagement.
Key Frontend Technologies
- To build responsive and interactive web applications, developers need to master:
- CSS (Cascading Style Sheets) – Styles web pages, ensuring a visually appealing layout.
Responsive Web Design
- With the growing use of mobile devices, developers must implement responsive design using:
- CSS Flexbox & Grid – For flexible layouts.
- Bootstrap & Tailwind CSS – Popular CSS frameworks for responsive design.
- A well-structured frontend improves user experience (UX) and ensures a consistent look across different devices.
4. Proficiency in JavaScript and Frameworks
Importance of JavaScript in Full Stack Development
JavaScript is the backbone of modern web applications. It enables dynamic content updates, form validation, and animations.
Popular JavaScript Frameworks
- Python Full Stack Developers should be familiar with JavaScript frameworks like:
- React.js – A component-based framework for building fast and scalable UIs.
- Angular.js – A full-fledged framework for developing enterprise-grade applications.
- Vue.js – A lightweight, flexible framework for modern web development.
Enhancing UI/UX with JavaScript
- DOM Manipulation – JavaScript modifies web page elements dynamically.
- AJAX & Fetch API – Enables data exchange between the frontend and backend without page reloads.
- State Management – Libraries like Redux (React) help manage application state efficiently.
- Mastering JavaScript alongside Python allows developers to build fully interactive applications.
5. Mastering Python for Backend Development
The backend is where Python shines, handling the logic and database interactions.
Why Python is Preferred for Backend Development
- Simplicity & Readability – Easy to write and understand.
- Robust Frameworks – Django and Flask speed up development.
- Scalability – Suitable for small to large-scale applications.
Key Python Backend Frameworks
- Python Full Stack Developers should master:
- Django – A high-level framework with built-in authentication, ORM, and security.
- Flask – A lightweight framework offering flexibility for smaller projects.
- FastAPI – Optimized for building high-performance APIs.
Writing Efficient and Scalable Python Code
- Use asynchronous programming (async/await) to handle multiple requests.
- Optimize database queries to reduce load times.
- Implement caching (Redis, Memcached) for improved performance.
- A strong backend ensures data integrity, security, and scalability.
6. Database Management Skills
- A Python Full Stack Developer must understand how to store, retrieve, and manipulate data efficiently. Databases are the backbone of web applications, storing user information, transactions, and system logs.
Types of Databases: SQL vs. NoSQL
- Databases fall into two main categories:
- Relational Databases (SQL) – Data is structured in tables and follows relationships. Examples:
- MySQL – Widely used, open-source database.
- PostgreSQL – Advanced database with JSON support and scalability.
- SQLite – Lightweight database for small projects.
- NoSQL Databases – Data is stored in flexible formats like JSON or key-value pairs. Examples:
- MongoDB – A document-oriented database ideal for scalable applications.
- Redis – In-memory data store used for caching and real-time analytics.
- ORM (Object-Relational Mapping) and Its Importance
- Instead of writing raw SQL queries, developers use ORMs to interact with databases using Python objects. Popular ORMs include:
- Django ORM – Simplifies database management in Django applications.
- SQLAlchemy – A powerful and flexible ORM for Flask applications.
- Best Practices for Database Management
- Indexing – Speeds up queries by reducing search time.
- Data Normalization – Eliminates redundancy and ensures efficient storage.
- Backup & Recovery – Regular database backups prevent data loss.
- Mastering database management ensures data integrity, security, and optimized performance.
7. RESTful API Development
- A RESTful API (Representational State Transfer) allows the frontend and backend to communicate, enabling data exchange between systems.
Basics of RESTful API
- A well-designed API follows these principles:
- Resource-Based Structure – Data is accessed as “resources” (/users, /orders).
- HTTP Methods:
- GET – Retrieve data.
- POST – Create new data.
- PUT/PATCH – Update existing data.
- DELETE – Remove data.
- Implementing APIs using Django REST Framework (DRF)
- Django REST Framework (DRF) simplifies API development in Python. Features include:
- Serializers – Converts Python objects into JSON format.
- Authentication – Supports JWT, OAuth, and token-based authentication.
- ViewSets & Routers – Automates API endpoint creation.
- API Authentication and Security
- Token-Based Authentication – Uses JWT (JSON Web Token) for secure logins.
- OAuth2 – Enables third-party authentication (Google, Facebook).
- Rate Limiting – Prevents API misuse by limiting requests per minute.
- Building secure and efficient APIs ensures smooth communication between the frontend and backend.
8. Version Control Systems (Git & GitHub)
- Version control helps developers track changes, collaborate, and maintain project history.
Why Version Control is Essential
- Tracks Code Changes – Prevents accidental data loss.
- Rollback Capability – Restores previous versions if necessary.
- Git Basics: Commits, Branching, Merging
- git init – Initializes a new Git repository.
- git add . – Stages files for commit.
- git branch – Creates and lists branches.
- git merge – Merges branches without conflicts.
- Using GitHub for Collaboration
- Developers use GitHub, GitLab, or Bitbucket to:
- Store and share repositories.
- Create pull requests for code review.
- Automate CI/CD pipelines for seamless deployment.
- Version control is a fundamental skill for maintaining clean, organized, and efficient codebases.
9. Understanding Web Servers and Deployment
- Once a web application is built, it needs to be deployed on a server to be accessible to users.
Role of Web Servers in Full Stack Development
- A web server processes HTTP requests and serves web pages to users. Common web servers include:
- Apache – Popular open-source web server.
- NGINX – Efficient and optimized for high traffic.
- Gunicorn – A Python WSGI server for running Django and Flask applications.
- Deployment Tools and Platforms
- Developers deploy applications using:
- Heroku – Simple cloud deployment with built-in database support.
- AWS (Amazon Web Services) – Provides scalable hosting solutions like EC2 and S3.
- Docker & Kubernetes – Helps containerize and manage applications efficiently.
- CI/CD Pipelines for Automated Deployment
- Continuous Integration and Deployment (CI/CD) automates testing and releases. Popular tools include:
- Jenkins – Automates build and test processes.
- GitHub Actions – Runs workflows within GitHub.
- Travis CI – Ensures stable releases with automated testing.
- A strong grasp of web servers and deployment ensures high availability and performance.
10.Security Best Practices for Web Applications
- Common Web Security Threats
Web applications are vulnerable to:
- SQL Injection – Attackers manipulate SQL queries to gain access to databases.
- Cross-Site Scripting (XSS) – Malicious scripts injected into web pages.
- Implementing Authentication & Authorization
- To secure user data, developers use:
- Secure Password Hashing – Use bcrypt or Argon2 for password storage.
- Role-Based Access Control (RBAC) – Restricts user permissions.
- Securing APIs and Data Encryption
- HTTPS (SSL/TLS) – Encrypts data transmission between client and server.
- API Rate Limiting – Prevents brute-force attacks.
- CORS (Cross-Origin Resource Sharing) – Restricts API access to trusted domains.
- Following security best practices protects applications from cyber threats.
11.Cloud Computing and Hosting
- Why Cloud Computing is Important
- Cloud computing eliminates the need for traditional on-premise servers and offers:
- Scalability – Applications handle traffic spikes efficiently.
- Global Access – Applications are available worldwide.
- Cloud Service Providers (AWS, GCP, Azure)
- Popular cloud platforms include:
- Amazon Web Services (AWS) – Offers services like EC2, S3, and RDS.
- Google Cloud Platform (GCP) – Provides scalable computing and AI services.
- Microsoft Azure – Supports web hosting, databases, and cloud storage.
- Deploying Python Applications in the Cloud
- Developers deploy applications using:
- AWS Elastic Beanstalk – Simplifies deployment for Python applications.
- Google App Engine – Deploys scalable web applications effortlessly.
- Docker & Kubernetes – Containerize and manage applications efficiently.
- Cloud computing ensures high availability, reliability, and performance.
12. DevOps and CI/CD Pipelines
- In modern web development, DevOps plays a critical role in automating processes, improving collaboration between development and operations teams, and ensuring reliable software delivery. A Python Full Stack Developer must understand the principles of Continuous Integration (CI) and Continuous Deployment (CD) to streamline development workflows.
Understanding DevOps Principles
- Continuous Integration (CI) – Developers frequently merge code changes into a shared repository to detect and fix issues early.
- Continuous Deployment (CD) – Automates the release process, allowing updates to be deployed without manual intervention.
- Infrastructure as Code (IaC) – Infrastructure configuration is managed through scripts and automation rather than manual setup.
- CI/CD Tools for Automation
- Python developers rely on the following tools to implement DevOps workflows:
- Jenkins – Automates builds, testing, and deployments.
- GitHub Actions – Runs automated workflows directly within GitHub.
- GitLab CI/CD – Integrates CI/CD pipelines within Git repositories.
- Monitoring and Logging Tools
- Monitoring and logging help in maintaining system reliability:
- Prometheus – Collects and stores real-time metrics.
- Grafana – Visualizes server performance and logs.
- Implementing DevOps practices improves efficiency, minimizes deployment failures, and enhances software reliability.
13. Soft Skills for Python Full Stack Developers
- Technical skills alone are not enough to succeed as a Python Full Stack Developer. Problem-Solving and Critical Thinking
- Developers must think logically to debug complex issues and optimize application performance.
- Ability to analyze problems and propose efficient solutions is a crucial skill in full stack development.
Communication and Collaboration
- Working with designers, product managers, and other developers requires clear and concise communication.
- Writing clean documentation helps ensure easy project handover.
- Time Management and Adaptability
- Developers often work on multiple projects simultaneously, so managing time effectively is key.
- The tech industry evolves rapidly, and staying adaptable to new frameworks and tools is crucial for career growth.
- A Python Full Stack Developer with strong soft skills is more productive, efficient, and valuable to any team.
14. Debugging and Testing Techniques
- Bugs and errors are inevitable in software development. Knowing how to debug and test efficiently is an essential skill for a Python Full Stack Developer.
Debugging Strategies for Python Applications
- Print Statements – A simple yet effective way to check variable values during execution.
- Logging – Using Python’s logging module to track errors and execution flow.
- Debugger Tools – Python’s built-in PDB (Python Debugger) allows developers to set breakpoints and inspect code execution.
- Unit Testing and Test-Driven Development (TDD)
- Unit Testing – Testing individual functions and components to ensure they work correctly.
- TDD Approach – Writing tests before writing actual code to guarantee accuracy.
- Automated Testing Frameworks
- Selenium – Automates browser testing for frontend applications.
- PyTest – A powerful Python testing framework for unit and functional tests.
- Jest – A JavaScript testing framework used for frontend testing in React and Angular.
- Effective debugging and testing prevent software failures, security vulnerabilities, and performance issues.
15. WebSockets and Real-Time Applications
- Traditional web applications use HTTP requests, but WebSockets enable real-time communication, essential for applications like live chats, notifications, and streaming services.
What are WebSockets?
- Unlike traditional request-response models, WebSockets maintain an always-open connection between the client and server.
- This enables instant data updates without needing to refresh the page.
- Implementing Real-Time Features with Django Channels
- Django Channels extends Django’s capabilities to support WebSockets.
- Used for real-time messaging apps, notifications, and live updates.
- Real-World Use Cases
- Messaging Applications – WhatsApp, Slack, and Discord use Web Sockets for real-time chat.
- Live Streaming Platforms – Twitch and YouTube Live rely on Web Sockets to update user interactions instantly.
- Stock Market Updates – Real-time financial dashboards use Web Sockets for live price tracking.
- Mastering Web Sockets allows developers to build interactive and dynamic web applications.
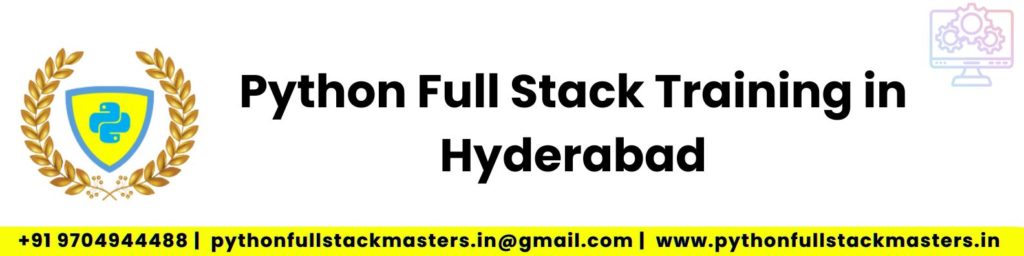
16. Building Scalable Applications
- Scalability ensures that web applications can handle increasing user traffic and data loads without performance degradation.
Why Scalability is Important
- Applications must handle high traffic without crashing.
- Load Balancing and Caching Mechanisms
- Load Balancers (NGINX, HAProxy) distribute traffic efficiently across multiple servers.
- Caching (Redis, Memcached) stores frequently accessed data to reduce database load.
- Handling High Traffic Efficiently
- Database Sharding – Splits large databases into smaller, manageable parts.
- Asynchronous Processing (Celery, RabbitMQ) – Handles background tasks efficiently.
- A well-architected application can scale seamlessly as user demands grow.
17. Understanding Microservices Architecture
- Many companies are shifting from monolithic applications to microservices to improve flexibility and maintainability.
Introduction to Microservices
- How Python Facilitates Microservices Development
- FastAPI – Ideal for lightweight microservices-based APIs.
- Flask – Frequently used for building microservices in Python-based ecosystems.
- Benefits Over Monolithic Architecture
- Fault Isolation – If one service fails, the rest of the application remains functional.
- Understanding microservices allows developers to build flexible, scalable, and modern applications.
18. Data Structures and Algorithms
- Strong knowledge of data structures and algorithms (DSA) improves problem-solving and coding efficiency.
Why Data Structures Matter for Developers
- Optimized algorithms lead to faster applications.
- Efficient searching and sorting techniques improve database queries and API performance.
- Key Algorithms and Their Applications
- Searching Algorithms – Binary Search, Hashing.
- Graph Algorithms – Dijkstra’s Algorithm (used in Google Maps).
- Python Libraries for Data Structures
- NumPy & Pandas – Handle large datasets efficiently.
- Collections Module – Offers advanced data structures like deque, defaultdict.
- A strong foundation in DSA gives developers an advantage in coding interviews and real-world applications.
19. Continuous Learning and Staying Updated
- The tech industry evolves rapidly, requiring developers to continuously learn and adapt.
- Best Resources for Learning New Technologies
- Online Courses – Udemy, Coursera, freeCodeCamp.
- Documentation & Blogs – Real Python, CSS Tricks.
- Tech Communities – Stack Overflow, GitHub, Reddit.
- Contributing to Open Source Projects
- Builds real-world experience.
- Enhances credibility as a developer.
20. Conclusion & Career Path
- Becoming a Python Full Stack Developer requires mastering frontend and backend technologies, databases, security, DevOps, and scalability.
- Career Opportunities
- Web Developer
- Software Engineer
- DevOps Engineer
- Cloud Architect
FAQS
1. What is a Python Full Stack Developer?
A developer who works on both frontend (UI) and backend (server, database) using Python (Django, Flask) and frontend technologies like HTML, CSS, JavaScript (React, Vue.js).
2. Which frameworks should I learn?
Backend: Django, Flask, FastAPI
Frontend: React.js, Vue.js, Angular
3. Is Python Full Stack Development a good career?
Yes! It offers high demand, good salaries, and remote work opportunities.
4. How long does it take to become one?
Beginners: 6-12 months
Intermediate: 3-6 months
Experienced: 1-3 months
5. Essential skills for a Python Full Stack Developer?
Frontend: HTML, CSS, JavaScript
Backend: Python, Django, Flask
Database: MySQL, PostgreSQL, MongoDB
APIs, Git, Deployment
6. Common challenges?
Keeping up with new technologies
Debugging frontend-backend integration
Security vulnerabilities
7. How much do they earn?
Entry-Level: $60k – $80k
Mid-Level: $80k – $120k
Senior: $120k+
8. Do I need a degree?
No, but a CS degree helps. Self-learning via online courses & projects is common.
9. Best beginner projects?
Portfolio Website
E-commerce Site
Blog System
Chat App (WebSockets)
10. How to stay updated?
Follow blogs (Real Python, Dev.to)
Take online courses (Udemy, Coursera)
Join communities (GitHub, Stack Overflow)
The Python Full Stack Training is a 2 months program offering online and offline classes. We covers Python fundamentals, front-end technologies like HTML training, CSS training, and React training and back-end frameworks such as Django and Flask. With hands-on project , expert guidance and free batch access until get placement."