Table of Contents
TogglePython Questions for Interview
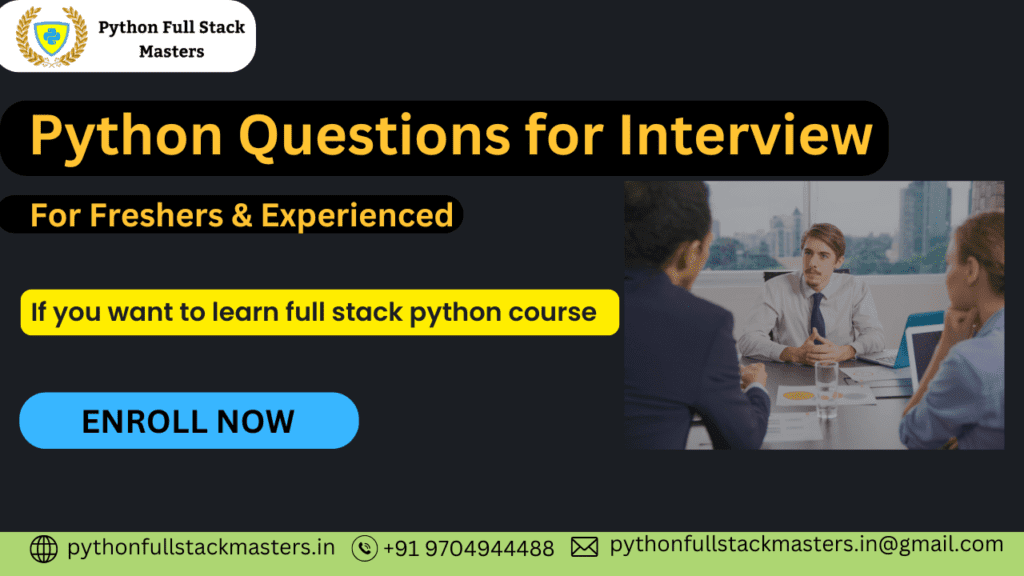
If you’re aiming to crack a Python interview, I’ve covered a list of commonly asked questions for different experience levels.
For Freshers (0-1 Year Experience): I have included fundamental Python questions to help you get started with the basics in a simple and easy-to-understand way.
For 2-3 Years of Experience: You’ll find intermediate-level questions that test your practical knowledge and problem-solving skills.
For 4-5 Years of Experience: I’ve added questions that focus on advanced concepts, performance optimization, and real-world applications.
For 10+ Years of Experience: You’ll find high-level questions covering system design, scalability, and best practices for enterprise applications.
Topics covered:
Python Interview Questions for Freshers
1. What is Python?
- Python is a high-level, interpreted programming language known for its simplicity and readability. It is widely used in web development, data science, automation, AI, and more.
2. What are the key features of Python?
- Easy to Learn & Readable – Uses simple syntax similar to English.
- Interpreted Language – Runs code line by line without compilation.
- Dynamically Typed – No need to declare variable types.
- Object-Oriented – Supports classes and objects.
- Extensive Libraries – Comes with many built-in modules and third-party libraries.
- Cross-Platform – Works on Windows, Mac, and Linux.
3. How is Python interpreted?
- Python code is executed line by line by the Python interpreter, which means you don’t need to compile it before running.
4. What is PEP 8?
- PEP 8 is Python’s official style guide that defines best practices for writing clean, readable, and consistent Python code. It includes rules for indentation, naming conventions, and formatting.
5. What are Python’s built-in data types?
- Numeric – int (whole numbers), float (decimals), complex (complex numbers).
- Text – str (string).
- Sequence – list (ordered, mutable), tuple (ordered, immutable), range.
- Set – set (unordered, unique elements).
- Mapping – dict (key-value pairs).
- Boolean – bool (True or False).
6. How do you comment in Python?
- Single-line comment – Use # before a line.
# This is a comment - Multi-line comment – Use triple quotes ”’ or “””.
“””This is a multi-line comment”””
7. What is indentation in Python?
- Indentation (spaces or tabs) is used to structure code blocks instead of using {} like in other languages.
Example:
if True:
print(“Indented code runs inside the block”)
- Incorrect indentation will cause an error.
8. How do you declare variables in Python?
- You simply assign a value without specifying a type:
x = 10 # Integer
name = “Alice” # String
price = 99.99 # Float
Python automatically detects the type.
9. How do you take user input in Python?
Use the input() function:
name = input(“Enter your name: “)
print(“Hello,”, name)
The input is always a string, so you may need to convert it:
age = int(input(“Enter your age: “)) # Convert to integer
10. What is the difference between is and ==?
- == compares values (checks if two variables have the same content).
- is compares object identity (checks if two variables point to the same memory location).
Example:
a = [1, 2, 3]
b = [1, 2, 3]
print(a == b) # True (because values are the same)
print(a is b) # False (because they are different objects in memory)
11. What is the difference between list and tuple?
- List: Mutable (can be changed), defined using [].
- Tuple: Immutable (cannot be changed), defined using ().
Example:
my_list = [1, 2, 3] # Can be modified
my_tuple = (1, 2, 3) # Cannot be modified
12. What is slicing in Python?
Slicing is used to extract a portion of a sequence (like lists, strings, tuples) using : notation.
Example:
my_list = [10, 20, 30, 40, 50]
print(my_list[1:4]) # Output: [20, 30, 40]
Syntax: sequence[start:end:step]
13. What are Python’s control structures?
Control structures manage the flow of execution in a program.
- Conditional statements: if, if-else, if-elif-else.
- Loops: for, while.
- Loop control statements: break, continue, pass.
Example:
x = 10
if x > 5:
print(“x is greater than 5”)
14. How do you create a function in Python?
Use the def keyword.
Example:
def greet(name):
print(“Hello,”, name)
greet(“Alice”) # Output: Hello, Alice
15. What is recursion?
Recursion is when a function calls itself to solve a problem.
Example:
def factorial(n):
if n == 0:
return 1
return n * factorial(n – 1)
print(factorial(5)) # Output: 120
It is used for problems like factorial, Fibonacci series, etc.
16. What is a lambda function?
A lambda function is a small, anonymous function that can have multiple arguments but only one expression.
Example:
square = lambda x: x * x
print(square(5)) # Output: 25
Equivalent to:
def square(x):
return x * x
17. What is a dictionary in Python?
A dictionary is a collection of key-value pairs. Defined using {}.
Example:
student = {“name”: “Alice”, “age”: 20}
print(student[“name”]) # Output: Alice
18. How do you handle exceptions in Python?
Use try-except to catch and handle errors.
Example:
try:
x = 10 / 0
except ZeroDivisionError:
print(“Cannot divide by zero”)
19. What is the difference between break and continue?
- break: Exits the loop completely.
- continue: Skips the current iteration and moves to the next.
Example:
for i in range(5):
if i == 3:
break # Stops loop at 3
print(i)
for i in range(5):
if i == 3:
continue # Skips 3
print(i)
20. What is a Python module?
- A module is a file containing Python code (functions, classes, variables) that can be imported and reused.
Example:
Creating a module (math_utils.py):
def add(a, b):
return a + b
Importing and using it:
import math_utils
print(math_utils.add(2, 3)) # Output: 5
21. What are Python packages?
- A package is a collection of modules (Python files) grouped together in a directory with an __init__.py file. It helps organize code.
Example:
mypackage/
│– __init__.py
│– module1.py
│– module2.py
Usage:
import mypackage.module1
22. What is the difference between a shallow copy and a deep copy?
- Shallow Copy: Copies only references to objects (changes affect both).
- Deep Copy: Copies everything, creating independent objects.
Example:
import copy
list1 = [[1, 2], [3, 4]]
shallow = copy.copy(list1) # Shares inner lists
deep = copy.deepcopy(list1) # Creates new copies of inner lists
list1[0][0] = 99
print(shallow[0][0]) # 99 (affected)
print(deep[0][0]) # 1 (unchanged)
23. What is the use of the range() function?
- The range() function generates a sequence of numbers.
Example:
for i in range(5): # 0 to 4
print(i)
It can also define a start and step:
for i in range(1, 10, 2): # 1 to 9, step 2
print(i)
24. How does Python handle memory management?
Python manages memory using:
- Automatic Garbage Collection – Removes unused objects.
- Reference Counting – Objects are deleted when no references exist.
- Memory Pools – Manages small objects efficiently.
Example:
import gc
gc.collect() # Manually trigger garbage collection
25. What are args and kwargs in Python?
They allow flexible function arguments.
- *args – Accepts multiple positional arguments.
- **kwargs – Accepts multiple keyword arguments.
Example:
def func(*args, **kwargs):
print(args) # Tuple of values
print(kwargs) # Dictionary of key-value pairs
func(1, 2, 3, name=”Alice”, age=25)
26. What is the difference between mutable and immutable data types?
- Mutable – Can be changed after creation (list, dict, set).
- Immutable – Cannot be changed (int, float, str, tuple).
Example:
x = “hello”
x[0] = “H” # ❌ Error (immutable)
lst = [1, 2, 3]
lst[0] = 100 # ✅ Works (mutable)
27. How do you reverse a string in Python?
Use slicing:
s = “Python”
print(s[::-1]) # Output: nohtyP
Or use reversed():
print(“”.join(reversed(s)))
28. What are Python’s built-in functions?
Python provides many built-in functions, such as:
- print() – Displays output
- len() – Returns length
- type() – Shows data type
- sorted() – Sorts elements
- sum() – Adds numbers
- map(), filter(), zip(), etc.
Example:
print(len(“hello”)) # Output: 5
print(type(10)) # Output: <class ‘int’>
29. How do you create a class in Python?
Use the class keyword.
Example:
class Car:
def __init__(self, brand):
self.brand = brand
def show(self):
print(“Car brand:”, self.brand)
c = Car(“Toyota”)
c.show()
30. What is the difference between instance, static, and class methods?
- Instance Method (self) – Works with object data.
- Class Method (cls) – Works with class-level data.
- Static Method – Independent of class and instance.
Example:
class Example:
def instance_method(self): # Instance method
print(“Instance method”)
@classmethod
def class_method(cls): # Class method
print(“Class method”)
@staticmethod
def static_method(): # Static method
print(“Static method”)
e = Example()
e.instance_method()
Example.class_method()
Example.static_method()
31. What is the use of the self keyword in Python?
- self represents the current instance of a class.
- It is used to access instance variables and methods inside a class.
Example:
class Person:
def __init__(self, name):
self.name = name # ‘self.name’ refers to instance variable
def greet(self):
print(“Hello, my name is”, self.name)
p = Person(“Alice”)
p.greet() # Output: Hello, my name is Alice
32. What is inheritance in Python?
- Inheritance allows a class (child) to use properties and methods of another class (parent).
- It helps in code reusability.
Example:
class Animal:
def speak(self):
print(“Animal speaks”)
class Dog(Animal): # Dog inherits from Animal
def bark(self):
print(“Dog barks”)
d = Dog()
d.speak() # Inherited method
d.bark() # Own method
33. What is polymorphism in Python?
- Polymorphism means one function or method can work in different ways depending on the object.
- It allows different classes to have methods with the same name but different behavior.
Example:
class Cat:
def speak(self):
return “Meow”
class Dog:
def speak(self):
return “Bark”
def animal_sound(animal):
print(animal.speak())
animal_sound(Cat()) # Output: Meow
animal_sound(Dog()) # Output: Bark
34. What is method overloading and method overriding?
- Method Overloading: Having multiple methods with the same name but different parameters (not directly supported in Python).
- Method Overriding: When a child class redefines a method from the parent class.
Example of method overriding:
class Parent:
def show(self):
print(“Parent method”)
class Child(Parent):
def show(self): # Overriding parent method
print(“Child method”)
c = Child()
c.show() # Output: Child method
35. How do you check the type of a variable in Python?
Use the type() function.
Example:
x = 10
print(type(x)) # Output: <class ‘int’>
y = [1, 2, 3]
print(type(y)) # Output: <class ‘list’>
36. What are Python’s file handling operations?
Python allows reading and writing files using built-in functions.
Common operations:
- open() – Open a file
- read() – Read contents
- write() – Write to a file
- close() – Close the file
Example:
file = open(“test.txt”, “w”) # Open file in write mode
file.write(“Hello, World!”) # Write text
file.close() # Close file
36. What are Python’s file handling operations?
Python allows reading and writing files using built-in functions.
Common operations:
- open() – Open a file
- read() – Read contents
- write() – Write to a file
- close() – Close the file
Example:
file = open(“test.txt”, “w”) # Open file in write mode
file.write(“Hello, World!”) # Write text
file.close() # Close file
37. What is the use of the with statement in file handling?
- The with statement automatically closes the file after use.
- It prevents memory leaks and errors.
Example:
with open(“test.txt”, “r”) as file:
data = file.read() # No need to call file.close()
print(data)
38. How do you read and write a file in Python?
Writing to a file:
with open(“example.txt”, “w”) as file:
file.write(“Hello, Python!”)
Reading a file:
with open(“example.txt”, “r”) as file:
content = file.read()
print(content)
39. What is a Python decorator?
- A decorator is a function that modifies another function’s behavior without changing its code.
- It is used for logging, authentication, etc.
Example:
def decorator(func):
def wrapper():
print(“Before function call”)
func()
print(“After function call”)
return wrapper
@decorator
def greet():
print(“Hello!”)
greet()
Output:
Before function call
Hello!
After function call
40. How do you work with JSON in Python?
Python provides the json module to read and write JSON data.
Convert dictionary to JSON (Serialization):
import json
data = {“name”: “Alice”, “age”: 25}
json_data = json.dumps(data) # Convert dictionary to JSON string
print(json_data) # Output: {“name”: “Alice”, “age”: 25}
Convert JSON to dictionary (Deserialization):
json_str = ‘{“name”: “Alice”, “age”: 25}’
dict_data = json.loads(json_str) # Convert JSON string to dictionary
print(dict_data[“name”]) # Output: Alice
Python Interview Questions For 2&3 Years Experience
1. What is the difference between pop() and remove() methods in lists?
- pop(index): Removes and returns an element at a specific index (default is the last item).
- remove(value): Removes the first occurrence of a specific value.
Example:
my_list = [1, 2, 3, 4, 5]
print(my_list.pop(2)) # Removes and returns 3
print(my_list) # Output: [1, 2, 4, 5]
my_list.remove(4) # Removes first occurrence of 4
print(my_list) # Output: [1, 2, 5]
2. What is the difference between append() and extend() methods in lists?
- append(value): Adds a single element to the list.
- extend(iterable): Adds multiple elements from an iterable (like another list).
Example:
my_list = [1, 2, 3]
my_list.append([4, 5])
print(my_list) # Output: [1, 2, 3, [4, 5]]
my_list = [1, 2, 3]
my_list.extend([4, 5])
print(my_list) # Output: [1, 2, 3, 4, 5]
3. What are Python’s string methods?
Some commonly used string methods:
- upper() – Converts to uppercase.
- lower() – Converts to lowercase.
- strip() – Removes leading and trailing spaces.
- replace(old, new) – Replaces a substring.
- find(substring) – Finds the index of a substring.
- startswith(prefix) – Checks if string starts with a prefix.
- endswith(suffix) – Checks if string ends with a suffix.
Example:
s = ” Hello, Python! “
print(s.strip()) # Output: “Hello, Python!”
print(s.replace(“Python”, “World”)) # Output: ” Hello, world.
4. How do you split and join strings in Python?
- split(delimiter): Splits a string into a list.
- join(iterable): Joins list elements into a string.
Example:
s = “apple,banana,grape”
fruits = s.split(“,”) # Convert to list
print(fruits) # Output: [‘apple’, ‘banana’, ‘grape’]
new_s = “-“.join(fruits) # Convert list to string
print(new_s) # Output: “apple-banana-grape”
5. What is a set in Python?
A set is an unordered collection of unique elements.
- Defined using {} or set().
- No duplicate values allowed.
Example:
my_set = {1, 2, 3, 3, 4}
print(my_set) # Output: {1, 2, 3, 4}
my_set.add(5)
print(my_set) # Output: {1, 2, 3, 4, 5}
6. How do you remove duplicates from a list in Python?
Convert the list to a set, then back to a list.
Example:
my_list = [1, 2, 2, 3, 4, 4, 5]
unique_list = list(set(my_list))
print(unique_list) # Output: [1, 2, 3, 4, 5]
7. What is the difference between sorted() and sort()?
- sorted(): Returns a new sorted list (does not modify original).
- sort(): Sorts the list in-place (modifies the original list).
Example:
numbers = [5, 2, 9, 1]
print(sorted(numbers)) # Output: [1, 2, 5, 9] (original list unchanged)
print(numbers) # Output: [5, 2, 9, 1]
numbers.sort()
print(numbers) # Output: [1, 2, 5, 9] (original list modified)
8. What are list comprehensions?
A concise way to create lists using a single line of code.
Example:
squares = [x*x for x in range(5)]
print(squares) # Output: [0, 1, 4, 9, 16]
Equivalent to:
squares = []
for x in range(5):
squares.append(x*x)
9. How do you install third-party packages in Python?
Use the pip package manager.
Example:
pip install requests
To check installed packages:
pip list
10. What is virtualenv in Python?
virtualenv is used to create isolated environments for Python projects.
This helps avoid conflicts between package versions.
Create a virtual environment:
python -m venv myenv
Activate the environment:
Windows:
myenv\Scripts\activate
Mac/Linux:
source myenv/bin/activate
To deactivate:
deactivate
11. What is Python’s Global Interpreter Lock (GIL)?
The Global Interpreter Lock (GIL) is a mechanism in CPython (the most common Python implementation) that allows only one thread to execute Python code at a time. This means that even if you use multiple threads, they won’t run Python code in parallel.
🔹 Why does Python have a GIL?
- It simplifies memory management and prevents race conditions.
- However, it limits the performance of multi-threaded CPU-bound tasks.
🔹 How to bypass GIL?
- Use the multiprocessing module instead of threading.
Example:
import threading
def task():
for _ in range(5):
print(“Running…”)
t1 = threading.Thread(target=task)
t2 = threading.Thread(target=task)
t1.start()
t2.start()
t1.join()
t2.join()
Even with multiple threads, Python executes only one at a time due to the GIL.
12. What are Python generators?
Generators are a way to create iterators in Python using the yield keyword.
Why use generators?
- They save memory (useful for large data).
- They produce values on demand instead of storing everything in memory.
Example:
def my_generator():
yield 1
yield 2
yield 3
gen = my_generator()
print(next(gen)) # Output: 1
print(next(gen)) # Output: 2
13. How does Python handle memory management?
Python has automatic memory management, which includes:
- Reference Counting – Python keeps track of how many references an object has. When an object’s reference count becomes zero, it is deleted.
- Garbage Collection – Python periodically removes unreachable objects.
Example:
a = [1, 2, 3]
b = a # Reference count increases
del a # Reference count decreases
Python’s garbage collector will free memory when a is no longer used.
14. What are metaclasses in Python?
A metaclass is a class that defines how other classes are created.
🔹 Normal classes create objects, but metaclasses create classes.
Example:
class MyMeta(type):
def __new__(cls, name, bases, dct):
print(f”Creating class {name}”)
return super().__new__(cls, name, bases, dct)
class MyClass(metaclass=MyMeta):
pass # When this class is defined, MyMeta’s __new__ runs
Output:
Creating class MyClass
Metaclasses are rarely needed but are useful for custom class behaviors.
15. What is the difference between __init__ and __new__?
- __new__ creates a new instance of the class.
- __init__ initializes the instance after creation.
Example:
class Example:
def __new__(cls):
print(“Creating instance”)
return super().__new__(cls)
def __init__(self):
print(“Initializing instance”)
obj = Example()
Output:
Creating instance
Initializing instance
When to use __new__?
- When modifying instance creation (e.g., for singleton patterns).
16. What is the difference between @staticmethod and @classmethod?
- @staticmethod – Belongs to the class, but does not access class or instance data.
- @classmethod – Takes cls as the first parameter and can modify class variables.
Example:
class Example:
class_var = “Python”
@staticmethod
def static_method():
return “I am static”
@classmethod
def class_method(cls):
return cls.class_var # Can access class variable
print(Example.static_method()) # Output: I am static
print(Example.class_method()) # Output: Pytho
Use @staticmethod when you don’t need class/instance data.
Use @classmethod when you need to modify class variables
17. How do you optimize Python code for performance?
Some ways to make Python code faster:
Use built-in functions – They are optimized in C.
sum([1, 2, 3, 4, 5]) # Faster than using a loop
Use list comprehensions – They are faster than loops.
squares = [x*x for x in range(10)]
Use generators – They save memory.
Use multiprocessing – To avoid the GIL for CPU-bound tasks.
Use caching – Use functools.lru_cache for expensive function calls.
Example:
from functools import lru_cache
@lru_cache(maxsize=100)
def expensive_function(n):
return n * n
18. What are Python’s threading and multiprocessing modules?
- Threading – Runs multiple threads in the same process (but affected by GIL).
- Multiprocessing – Runs multiple independent processes, each with its own memory.
Example (Threading):
import threading
def task():
print(“Thread running”)
t = threading.Thread(target=task)
t.start()
Example (Multiprocessing):
import multiprocessing
def task():
print(“Process running”)
p = multiprocessing.Process(target=task)
p.start()
Use multiprocessing for CPU-bound tasks (e.g., heavy calculations).
Use threading for I/O-bound tasks (e.g., file reading, web scraping).
19. What is the difference between multithreading and multiprocessing?
Feature | Multithreading | Multiprocessing |
Memory Usage | Shared memory | Separate memory |
Performance | Slower (GIL limit) | Faster for CPU-heavy tasks |
Best for | I/O-bound tasks (file I/O, web scraping) | CPU-bound tasks (heavy calculations) |
20. How does Python handle concurrency?
Python provides multiple ways to run tasks concurrently (overlapping execution):
✅ Threading – Runs multiple threads but affected by the GIL.
✅ Multiprocessing – Runs separate processes (best for CPU-heavy tasks).
✅ Asyncio – Best for handling I/O-bound tasks (e.g., making multiple API calls).
Example (Asyncio for concurrency):
import asyncio
async def task():
print(“Task started”)
await asyncio.sleep(2) # Simulates waiting
print(“Task finished”)
asyncio.run(task())
21. What is asynchronous programming in Python?
Asynchronous programming allows Python to handle multiple tasks at the same time without waiting for each task to finish. It is useful for I/O-bound tasks like downloading files, making API calls, or database operations.
🔹 Synchronous (Normal Execution) – Tasks run one after another, waiting for each to complete.
🔹 Asynchronous Execution – Tasks can run in the background without blocking the main program.
Example (Synchronous):
import time
def task():
print(“Task started”)
time.sleep(3) # Simulates a delay
print(“Task finished”)
task()
print(“Next task starts”)
Output (Waits for 3 seconds before moving to the next task):
Task started
Task finished
Next task starts
Example (Asynchronous):
import asyncio
async def task():
print(“Task started”)
await asyncio.sleep(3) # Doesn’t block other tasks
print(“Task finished”)
async def main():
await asyncio.gather(task(), task()) # Runs tasks in parallel
asyncio.run(main())
Output (Both tasks run at the same time):
Task started
Task started
(Task runs in parallel, waits 3 sec)
Task finished
Task finished
22. What is the async and await keyword in Python?
- async defines an asynchronous function that can run in the background.
- await pauses execution of the async function until the awaited task is done.
Example:
import asyncio
async def greet():
await asyncio.sleep(2) # Simulates a delay
print(“Hello, Async World!”)
asyncio.run(greet()) # Runs the async function
23. What is memoization in Python?
- Memoization is a technique to cache function results so that repeated calls with the same arguments don’t recompute the result, saving time.
- Python provides functools.lru_cache for memoization.
Example:
from functools import lru_cache
@lru_cache(maxsize=100) # Stores last 100 results
def fibonacci(n):
if n < 2:
return n
return fibonacci(n – 1) + fibonacci(n – 2)
print(fibonacci(10)) # Fast because previous values are cached
24. What are function annotations in Python?
Function annotations allow adding type hints to function parameters and return values.
Example:
def add(a: int, b: int) -> int:
return a + b
print(add(5, 10)) # Works normally
25. How do you use Python’s logging module?
The logging module is used to record debug messages, errors, or warnings in a structured way.
Example:
import logging
logging.basicConfig(level=logging.INFO) # Set logging level
logging.debug(“This is a debug message”)
logging.info(“This is an info message”)
logging.warning(“This is a warning message”)
logging.error(“This is an error message”)
26. What are Python’s design patterns?
Design patterns are reusable solutions to common programming problems.
🔹 Some common design patterns in Python:
- Singleton – Ensures only one instance of a class exists.
- Factory Pattern – Creates objects without specifying exact class.
- Observer Pattern – Used in event-driven programming.
Example (Factory Pattern):
class Car:
def drive(self):
return “Driving a car”
class Bike:
def ride(self):
return “Riding a bike”
def vehicle_factory(vehicle_type):
vehicles = {“car”: Car, “bike”: Bike}
return vehicles.get(vehicle_type, Car)() # Creates an object
v = vehicle_factory(“car”)
print(v.drive()) # Output: Driving a car
27. How do you implement the Singleton design pattern in Python?
Singleton ensures that only one instance of a class is created.
Example:
class Singleton:
_instance = None
def __new__(cls):
if cls._instance is None:
cls._instance = super().__new__(cls)
return cls._instance
obj1 = Singleton()
obj2 = Singleton()
print(obj1 is obj2) # Output: True (Both refer to the same instance)
28. What is the difference between duck typing and static typing?
- Duck Typing (Python’s approach) – The type of an object is determined by its behavior, not its explicit type.
- Static Typing (like in Java, C++) – The type of a variable is fixed at compile time.
Example of Duck Typing:
class Bird:
def fly(self):
print(“Flying”)
class Airplane:
def fly(self):
print(“Airplane flying”)
def take_off(obj):
obj.fly() # Works for both Bird and Airplane
take_off(Bird()) # Output: Flying
take_off(Airplane()) # Output: Airplane flying
29. How do you handle circular imports in Python?
Circular imports occur when two or more modules depend on each other.
🔹 Solutions:
- Use import inside a function to delay execution.
- Use import module_name instead of from module import something.
- Break dependency by moving common code to a third module.
Example (Fixing circular import using local import):
# module_a.py
def function_a():
from module_b import function_b # Local import avoids circular import
function_b()
# module_b.py
def function_b():
print(“Function B”)
30. What is the use of super() in Python?
The super() function allows calling a method from the parent class in a child class.
Example:
class Parent:
def greet(self):
print(“Hello from Parent”)
class Child(Parent):
def greet(self):
super().greet() # Calls the Parent method
print(“Hello from Child”)
c = Child()
c.greet()
Output:
Hello from Parent
Hello from Child
Python Interview Questions For 4&5 Years Experience
1. What is Monkey Patching in Python?
Monkey patching means changing a module or class at runtime, usually by modifying or replacing methods.
🔹 Example: Modifying a class method at runtime
class Person:
def greet(self):
return “Hello!”
# Define a new function
def new_greet():
return “Hi, I’m patched!”
# Apply monkey patch
Person.greet = new_greet
p = Person()
print(p.greet()) # Output: Hi, I’m patched!
2. What is the difference between deepcopy() and copy()?
Both are used to copy objects, but they behave differently:
- copy.copy() – Shallow Copy (only copies references, not nested objects)
- copy.deepcopy() – Deep Copy (creates a completely new object)
🔹 Example
import copy
original = [[1, 2, 3], [4, 5, 6]]
shallow = copy.copy(original)
deep = copy.deepcopy(original)
original[0][0] = 99
print(shallow) # [[99, 2, 3], [4, 5, 6]] (changes reflected)
print(deep) # [[1, 2, 3], [4, 5, 6]] (remains unchanged)
3. How does Python’s Garbage Collection work?
Python automatically manages memory using reference counting and garbage collection.
🔹 Memory Management Techniques:
- Reference Counting – An object is deleted when no references exist.
- Garbage Collector – Clears objects with circular references.
🔹 Example (Reference Counting)
import sys
a = [1, 2, 3]
print(sys.getrefcount(a)) # Output: 2 (itself + reference from getrefcount)
🔹 Example (Manually Running Garbage Collector)
import gc
gc.collect() # Forces garbage collection
4. What is the difference between a module and a package?
Feature | Module | Package |
What it is? | A single Python file (.py) | A collection of modules (folder with __init__.py) |
Example | math.py | numpy (contains multiple modules) |
Usage | import math | import numpy.random |
5. What are weakref in Python?
weakref allows referencing objects without preventing them from being garbage collected.
🔹 Use Case – Avoids preventing automatic memory cleanup.
🔹 Example
import weakref
class Example:
pass
obj = Example()
weak_obj = weakref.ref(obj)
print(weak_obj()) # Output: <__main__.Example object at 0x…>
del obj # Object is deleted
print(weak_obj()) # Output: None (object no longer exists)
6. How do you handle memory leaks in Python?
A memory leak happens when objects stay in memory but are no longer needed.
🔹 Ways to Prevent Memory Leaks:
- Use del to remove objects
- Use weak references (weakref)
- Avoid circular references
- Manually run garbage collection (gc.collect())
🔹 Example (Avoid Circular References)
import gc
class A:
def __init__(self):
self.ref = self # Circular reference
a = A()
del a # Object still in memory because of self-reference
gc.collect() # Forces cleanup
7. How do you profile Python code?
Profiling helps find slow parts of your program.
🔹 Using cProfile (Built-in Profiler)
python -m cProfile my_script.py
🔹 Using cProfile in Code
import cProfile
def test():
total = 0
for i in range(1000000):
total += i
return total
cProfile.run(‘test()’)
8. What are Python’s built-in optimization techniques?
Python has built-in ways to improve speed and efficiency:
Using List Comprehensions (Faster than loops)
squares = [x*x for x in range(10)] # Faster than using for-loop
Using Generators (Saves memory)
def generate_numbers():
for i in range(10):
yield i # Generates values on demand
gen = generate_numbers()
print(next(gen)) # Output: 0
Using set() for Fast Lookups
values = set([1, 2, 3])
print(3 in values) # Fast O(1) lookup
9. How do you use itertools in Python?
The itertools module provides efficient looping and iteration tools.
🔹 Examples of itertools:
import itertools
1. Infinite counter
counter = itertools.count(start=1, step=2)
print(next(counter)) # Output: 1
print(next(counter)) # Output: 3
2. Cycle through items
colors = itertools.cycle([“red”, “blue”])
print(next(colors)) # Output: red
print(next(colors)) # Output: blue
3. Combinations
letters = [“A”, “B”, “C”]
print(list(itertools.combinations(letters, 2))) # [(‘A’, ‘B’), (‘A’, ‘C’), (‘B’, ‘C’)]
10. How do you work with databases in Python?
Python provides SQLite, MySQL, PostgreSQL, and MongoDB support.
🔹 Example: SQLite with sqlite3
import sqlite3
# Connect to database (or create it)
conn = sqlite3.connect(‘test.db’)
cursor = conn.cursor()
# Create table
cursor.execute(“CREATE TABLE IF NOT EXISTS users (id INTEGER, name TEXT)”)
# Insert data
cursor.execute(“INSERT INTO users VALUES (1, ‘Alice’)”)
conn.commit()
# Fetch data
cursor.execute(“SELECT * FROM users”)
print(cursor.fetchall()) # Output: [(1, ‘Alice’)]
conn.close()
11. What is an ORM in Python?
ORM (Object-Relational Mapping) is a technique that lets you interact with a database using Python objects instead of writing raw SQL queries.
🔹 Popular ORMs in Python:
- SQLAlchemy
- Django ORM
- Peewee
🔹 Example: Using SQLAlchemy ORM
from sqlalchemy import create_engine, Column, Integer, String
from sqlalchemy.orm import declarative_base, sessionmaker
Base = declarative_base()
class User(Base):
__tablename__ = “users”
id = Column(Integer, primary_key=True)
name = Column(String)
engine = create_engine(“sqlite:///test.db”)
Base.metadata.create_all(engine)
12. What are Python’s frameworks for web development?
Python has several web frameworks, including:
- Flask – Lightweight and simple for small projects.
- Django – Full-featured and best for large applications.
- FastAPI – High-performance, used for APIs.
- Pyramid – Flexible and scalable.
- Tornado – Handles real-time applications well.
13. What is Flask, and how is it different from Django?
Feature | Flask | Django |
Type | Micro-framework | Full-featured framework |
Flexibility | More flexible | Less flexible (convention-based) |
Database Support | No built-in ORM | Built-in ORM |
Suitable for | Small projects & APIs | Large applications |
14. How do you handle API requests in Python?
You can handle API requests using the requests module.
🔹 Example: Sending an HTTP GET request
import requests
response = requests.get(“https://jsonplaceholder.typicode.com/todos/1”)
print(response.json()) # Output: JSON response
🔹 Example: Handling API in Flask
from flask import Flask, request, jsonify
app = Flask(__name__)
@app.route(“/api/data”, methods=[“GET”])
def get_data():
return jsonify({“message”: “Hello, API!”})
if __name__ == “__main__”:
app.run()
15. What is the difference between SOAP and REST APIs?
Feature | SOAP | REST |
Protocol | Uses XML | Uses JSON, XML |
Complexity | More complex | Simpler |
Speed | Slower | Faster |
Use Case | Enterprise apps (banking, payments) | Web & mobile apps |
16. How do you use WebSockets in Python?
WebSockets allow real-time communication between a client and a server.
🔹 Example: Using websockets library in Python
import asyncio
import websockets
async def server(websocket, path):
await websocket.send(“Hello, WebSocket!”)
message = await websocket.recv()
print(“Received:”, message)
start_server = websockets.serve(server, “localhost”, 8765)
asyncio.get_event_loop().run_until_complete(start_server)
asyncio.get_event_loop().run_forever()
17. What are Python’s testing frameworks?
Popular testing frameworks in Python:
- unittest – Built-in unit testing framework.
- pytest – More powerful and easier to use.
- nose2 – Similar to unittest but with better test discovery.
18. How do you write unit tests in Python?
Unit tests ensure that individual pieces of code work as expected.
🔹 Example: Using unittest
import unittest
def add(a, b):
return a + b
class TestMath(unittest.TestCase):
def test_add(self):
self.assertEqual(add(2, 3), 5)
if __name__ == “__main__”:
unittest.main()
19. How do you mock objects in Python?
Mocking is used in testing to simulate objects like databases or APIs.
🔹 Example: Using unittest.mock
from unittest.mock import MagicMock
class Database:
def get_data(self):
return “Real Data”
db = Database()
db.get_data = MagicMock(return_value=”Mocked Data”)
print(db.get_data()) # Output: Mocked Data
20. What is dependency injection in Python?
Dependency Injection (DI) is a design pattern where a class gets dependencies from outside rather than creating them internally.
🔹 Example: Without Dependency Injection
class Database:
def connect(self):
return “Connected to Database”
class Service:
def __init__(self):
self.db = Database() # Hardcoded dependency
def get_data(self):
return self.db.connect()
🔹 Example: With Dependency Injection
class Database:
def connect(self):
return “Connected to Database”
class Service:
def __init__(self, db):
self.db = db # Injecting dependency
def get_data(self):
return self.db.connect()
db_instance = Database()
service = Service(db_instance)
print(service.get_data()) # Output: Connected to Database
21. How do you containerize Python applications using Docker?
Containerization packages an application and its dependencies into a lightweight, portable container.
🔹 Steps to Containerize a Python App:
- Install Docker (docker –version to check).
- Create a Dockerfile to define the container image.
- Build and run the container.
🔹 Example: Dockerfile for a Flask App
# Use an official Python image
FROM python:3.9
# Set working directory in container
WORKDIR /app
# Copy project files
COPY . .
# Install dependencies
RUN pip install -r requirements.txt
# Run the application
CMD [“python”, “app.py”]
🔹 Commands to Build & Run the Container:
docker build -t my-python-app .
docker run -p 5000:5000 my-python-app
22. What are microservices, and how do they relate to Python?
Microservices are a way of breaking an application into smaller, independent services that communicate via APIs.
🔹 Microservices in Python:
- Flask & FastAPI – To build individual microservices.
- Docker & Kubernetes – To deploy microservices.
- RabbitMQ & Celery – For async communication between services.
23. How do you secure a Python web application?
To secure a Python web app:
- Use HTTPS – Encrypt data transmission.
- Sanitize user input – Prevent SQL injection & XSS.
- Implement authentication – Use JWT or OAuth.
- Protect against CSRF attacks – Use CSRF tokens.
- Limit API access – Use rate limiting.
- Keep dependencies updated – Check with pip list –outdated.
24. What is JWT authentication in Python?
JWT (JSON Web Token) is a secure way to handle authentication and authorization in web apps.
🔹 How JWT Works:
- User logs in → Server generates a JWT token.
- Token is sent to the client and stored (e.g., in local storage).
- Every API request includes the token.
- The server verifies the token before allowing access.
🔹 Example: Generating a JWT Token in Python
import jwt
payload = {“user_id”: 1}
secret_key = “mysecret”
token = jwt.encode(payload, secret_key, algorithm=”HS256″)
print(token) # Encrypted JWT token
25. How do you deploy Python applications?
Common ways to deploy Python apps:
- Using Gunicorn & Nginx (for Flask/Django)
- Docker & Kubernetes (for scalable deployments)
- Cloud Platforms – AWS, GCP, Azure, Heroku
- Serverless Deployment – AWS Lambda, Google Cloud Functions
🔹 Example: Deploying a Flask App with Gunicorn
gunicorn –bind 0.0.0.0:8000 app:app
26. What is the use of Celery in Python?
Celery is an asynchronous task queue that allows background job execution.
🔹 Use Cases:
- Processing emails.
- Handling long-running tasks.
- Running scheduled jobs.
🔹 Example: Using Celery with Flask
from celery import Celery
app = Celery(“tasks”, broker=”redis://localhost:6379/0″)
@app.task
def add(x, y):
return x + y
27. What are task queues in Python?
A task queue is used to handle background jobs asynchronously.
🔹 Popular Task Queues:
- Celery (with Redis/RabbitMQ)
- RQ (Redis Queue)
- Dramatiq
28. How do you work with large datasets in Python?
Handling large datasets requires memory-efficient techniques.
🔹 Best Practices:
- Use Pandas for structured data (read_csv() with chunksize).
- Use Dask for parallel computing.
- Use Generators (yield) to process data in chunks.
- Store data in databases instead of in-memory.
🔹 Example: Reading Large CSV in Chunks
import pandas as pd
for chunk in pd.read_csv(“large_file.csv”, chunksize=1000):
process(chunk) # Process data in batches
29. How do you implement caching in Python?
Caching stores frequently used data to improve performance.
🔹 Common Caching Techniques:
- Using functools.lru_cache (for function results).
- Redis/Memcached (for web applications).
🔹 Example: Using lru_cache to Cache Function Results
from functools import lru_cache
@lru_cache(maxsize=100)
def expensive_function(n):
return sum(range(n))
print(expensive_function(1000000)) # Cached after first ca
30. What are Python’s best practices for production environments?
- Use a Virtual Environment – Isolate dependencies with venv.
- Follow Logging Best Practices – Use logging instead of print().
- Implement Exception Handling – Avoid app crashes.
- Optimize Database Queries – Use indexing and caching.
- Keep Secrets Secure – Store API keys in environment variables.
- Use WSGI Servers – Deploy with Gunicorn for better performance.
- Enable Security Features – Use HTTPS, CSRF protection, and authentication.
- Monitor Performance – Use Prometheus or New Relic.
Python Interview Questions For 10 Years Experience
1. How do you design a scalable microservices architecture using Python?
- Microservices architecture involves breaking down an application into smaller, independent services. In Python, you can use Flask/FastAPI for lightweight services, Celery for task queues, Redis/Kafka for message brokering, and Docker/Kubernetes for containerization and deployment.
2. How do you handle real-time data processing in Python?
- Real-time data processing can be done using Apache Kafka, Celery, or Dask. Python libraries like Pandas and PySpark help process data efficiently.
3. What are the best practices for securing a Python-based enterprise application?
- Use HTTPS and authentication tokens (JWT, OAuth)
- Sanitize user input to prevent SQL Injection and XSS
- Implement rate limiting to prevent DDoS attacks
- Use secure coding practices and perform regular security audits
4. What is Python’s Global Interpreter Lock (GIL) and how do you handle it?
The GIL allows only one thread to execute at a time, limiting CPU-bound operations. To handle this, use multiprocessing instead of multithreading for parallel execution.
5. How do you optimize Python code for better performance?
- Use list comprehensions instead of loops
- Optimize memory usage with generators
- Use NumPy/Pandas for data processing
- Profile code with cProfile to find bottlenecks
6. What are Python’s asynchronous programming techniques?
Python supports async programming using the async and await keywords. Libraries like asyncio, FastAPI, and Celery help handle non-blocking tasks efficiently.
7. How do you implement caching in Python applications?
Use Redis, Memcached, or functools.lru_cache() to cache frequently accessed data and reduce load on databases.
8. What are some Python libraries used for big data processing?
- PySpark (Apache Spark for distributed computing)
- Dask (parallel computing)
- Pandas (data analysis)
9. How do you handle memory leaks in Python?
- Use weakref to avoid unnecessary object references
- Monitor memory usage with tracemalloc
- Free unused memory using gc.collect()
10. What is dependency injection in Python?
- Dependency Injection is a design pattern where objects receive their dependencies rather than creating them. In Python, frameworks like Flask-Injector help manage dependencies efficiently.
11. What is a design pattern, and how do you implement the Singleton pattern in Python?
- A design pattern is a reusable solution to a common problem. The Singleton pattern ensures only one instance of a class exists.
class Singleton:
_instance = None
def __new__(cls):
if cls._instance is None:
cls._instance = super().__new__(cls)
return cls._instance
12. How do you profile and optimize Python code?
- Use cProfile to measure execution time
- Use line_profiler for function profiling
- Optimize database queries with ORM tuning
13. How do you handle circular imports in Python?
- Use import inside a function instead of globally
- Refactor code to avoid circular dependencies
- Use sys.modules to check existing imports
14. What is the difference between SOAP and REST APIs?
- REST: Uses JSON, lightweight, faster
- SOAP: Uses XML, supports advanced security features
15. How do you containerize Python applications using Docker?
- Create a Dockerfile
- Use docker build to create an image
- Deploy using docker run or orchestrate with Kubernetes
16. How do you implement JWT authentication in Python?
- Use PyJWT to generate and validate JWT tokens for secure authentication in web applications.
17. What is Celery, and why is it used in Python?
- Celery is an asynchronous task queue that helps execute background tasks like sending emails, processing large datasets, and running scheduled jobs.
18. How do you manage configuration settings in Python applications?
- Use dotenv to store secrets
- Use configparser or environment variables
- Use AWS Secrets Manager for sensitive credentials
19. How do you mock objects in Python for unit testing?
Use the unittest.mock module to simulate objects and test code behavior without actual dependencies.
from unittest.mock import MagicMock
mock_obj = MagicMock()
mock_obj.method.return_value = “mocked”
20. What are Python’s best practices for production environments?
- Use virtual environments for dependency isolation
- Enable logging instead of print()
- Monitor application performance using Prometheus/Grafana
- Secure APIs with rate limiting and authentication
Additional Resources: