Table of Contents
TogglePython Practice Problems for Beginners
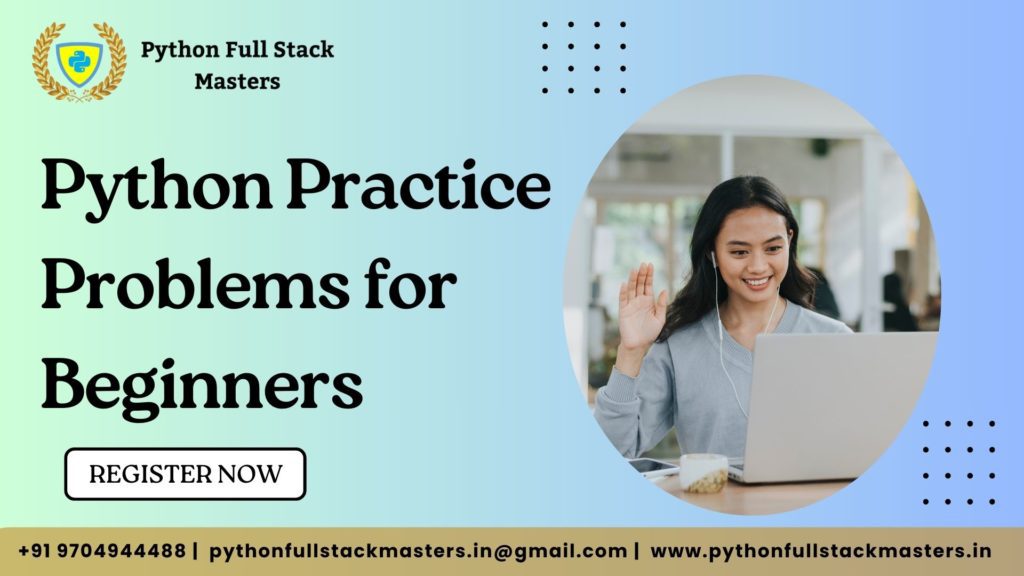
1.Introduction
It’s widely used in web development, data science, artificial intelligence, automation, and much more. If you’re just starting with Python, practicing small problems is a great way to strengthen your understanding of coding concepts.
Many beginners make the mistake of only reading about Python or watching tutorials without actually writing code. While learning theory is essential, hands-on practice is the key to mastering Python. When you solve problems, you develop logical thinking, learn debugging skills, and get comfortable with Python syntax.
This article will walk you through 20 practice problems, ranging from basic to slightly advanced. These problems will help you understand fundamental programming concepts like loops, conditionals, functions, recursion, and list manipulation. Each problem will be explained in detail, with different approaches for solving it.
By the end of this guide, you’ll gain confidence in writing Python programs and be ready to tackle more complex challenges. Whether you’re preparing for coding interviews, working on personal projects, or just honing your problem-solving skills, these exercises will set a strong foundation. So, let’s dive in.
2. Understanding Python Basics
Before jumping into the problems, let’s briefly go over some essential Python concepts that you need to be familiar with.
Variables and Data Types
In Python, variables store data values. Unlike other programming languages, you don’t need to declare a variable’s type explicitly.
python
x = 10 # Integer
y = 3.14 # Float
name = “John” # String
is_valid = True # Boolean
Understanding data types is crucial because Python treats them differently when performing operations.
Basic Input and Output
python
name = input(“Enter your name: “)
print(“Hello, ” + name + “!”)
User input is always read as a string, so conversion may be necessary:
python
age = int(input(“Enter your age: “)) # Converts string input to an integer
print(“Next year, you will be”, age + 1)
Comments and Indentation
Python uses indentation instead of curly braces {} to define blocks of code. This makes the code more readable but requires careful formatting.
python
if age >= 18:
print(“You are an adult.”) # Indented properly
Comments are essential for explaining your code.
python
# This is a single-line comment
“””
This is a multi-line comment
or a docstring.
“””
3. Problem 1: Printing "Hello, World!" Python Practice Problems for Beginners
Why This Problem?
The first step in any programming language is learning how to print output to the screen. This simple exercise helps beginners understand the print() function.
Solution and Explanation
The simplest way to print a message in Python is:
python
print(“Hello, World!”)
When you run this program, it displays:
Hello, World!
Variations of Printing Output
Printing multiple strings:
python
print(“Hello”, “World!”)
Output:
nginx
Hello World!
Using string concatenation:
python
print(“Hello” + ” ” + “World!”)
Using formatted strings (f-strings):
python
name = “Alice”
print(f”Hello, {name}!”)
Hands-on Practice
Try modifying the program to:
Print your own name instead of “World”
Print multiple lines using \n
Experiment with different ways of formatting
4. Problem 2: Simple Arithmetic Operations
name = “Alice”
print(f”Hello, {name}!”)
Hands-on Practice
Try modifying the program to:
Print your own name instead of “World”
Print multiple lines using \n
Experiment with different ways of formatting
Why This Problem?
Arithmetic operations form the foundation of programming logic. Understanding how to manipulate numbers is crucial for writing effective Python programs.
Solution and Explanation
Python supports basic arithmetic operations:
python
a = 10
b = 5
print(“Addition:”, a + b)
print(“Subtraction:”, a – b)
print(“Multiplication:”, a * b)
print(“Division:”, a / b)
Using input() for User Input
Let’s create a program that asks the user to enter two numbers and performs arithmetic operations:
python
num1 = float(input(“Enter first number: “))
num2 = float(input(“Enter second number: “))
print(“Sum:”, num1 + num2)
print(“Difference:”, num1 – num2)
print(“Product:”, num1 * num2)
print(“Quotient:”, num1 / num2)
Hands-on Practice
Modify the program to:
Add a modulus (%) operation
Round the results to two decimal places using round()
5. Problem 3: Swapping Two Variables
Why This Problem?
Swapping two variables is a classic problem that helps beginners understand assignment operations and memory management.
Solution and Explanation
The traditional method of swapping two variables uses a temporary variable:
python
a = 5
b = 10
temp = a
a = b
b = temp
Pythonic Approach: Tuple Unpacking
Real-World Applications
Swapping elements in sorting algorithms
Data structure manipulations
Hands-on Practice
Try swapping variables without using a temporary variable or tuple unpacking.
6. Problem 4: Checking Even or Odd Numbers
if a >= b and a >= c:Why This Problem?
Determining whether a number is even or odd is one of the first logic-based problems a beginner encounters. It introduces the modulus operator (%), conditional statements, and user input handling.
Solution and Explanation
Otherwise, it is odd.
python
num = int(input(“Enter a number: “))
if num % 2 == 0:
print(num, “is even.”)
else:
print(num, “is odd.”)
Understanding the Modulus Operator (%)
The modulus operator gives the remainder when one number is divided by another.
10 % 2 = 0 (Even)
15 % 2 = 1 (Odd)
Handling Edge Cases
What if the user enters 0? (It’s even.)
What about negative numbers? (Still works the same way.)
Hands-on Practice
Modify the program to check multiple numbers using a loop.
Print whether the number is positive, negative, or zero before checking even/odd.
7. Problem 5: Finding the Largest of Three Numbers
Why This Problem?
Comparing three numbers is a great way to understand conditional statements and logical comparisons.
Solution and Explanation
Using if-elif-else conditions:
a = float(input(“Enter first number: “))
b = float(input(“Enter second number: “))
c = float(input(“Enter third number: “))
print(“Largest number is:”, a)
elif b >= a and b >= c:
print(“Largest number is:”, b)
else:
print(“Largest number is:”, c)
Using Python’s max() Function
Python makes this easier with the built-in max() function:
python
print(“Largest number is:”, max(a, b, c))
Edge Cases and Testing
What if two or all numbers are the same?
How does it handle negative numbers?
Hands-on Practice
Modify the program to also find the smallest number using min().
8. Problem 6: Calculating the Factorial of a Number Why This Problem?
Factorials are widely used in mathematics and programming, especially in permutations, combinations, and recursion problems.
Solution and Explanation
Using a loop:
python
num = int(input(“Enter a number: “))
factorial = 1
if num < 0:
<mark id=”p_9″>print(“Factorial is not defined for negative numbers.”)
elif num == 0 or num == 1:
Using Recursion
num = int(input(“Enter a number: “))
print(“Factorial of”, num, “is”, factorial(num))
Hands-on Practice
Modify the program to find factorials of multiple numbers in a loop.
Implement the solution using Python’s built-in math.factorial() function.
9. Problem 7: Generating Fibonacci Series
Why This Problem?
The Fibonacci series is a famous sequence used in problem-solving and interviews. It helps understand loops, recursion, and sequence generation.
Solution and Explanation
The Fibonacci sequence starts as:
Using a loop:
python
n = int(input(“Enter the number of terms: “))
a, b = 0, 1
print(“Fibonacci sequence:”)
for _ in range(n):
print(a, end=” “)
Using Recursion
Recursion can generate the sequence but is less efficient for large values.
python
def fibonacci(n):
if n <= 1:
<mark id=”p_24″>return n
num_terms = int(input(“Enter number of terms: “))
for i in range(num_terms):
print(fibonacci(i), end=” “)
Hands-on Practice
Modify the loop version to store results in a list.
Optimize the recursive approach using memoization.
10. Problem 8: Checking Prime Numbers
Why This Problem?
Prime numbers play a crucial role in cryptography, mathematics, and computer science. Checking for primes efficiently is an important skill.
Solution and Explanation
Using a loop:
python
num = int(input(“Enter a number: “))
Optimizing the Algorithm
Instead of checking all numbers up to n, we check up to √n.
If a number has a divisor larger than √n, it must also have a smaller divisor.
Edge Cases
1 is not prime.
Negative numbers are not prime.
2 is the only even prime number.
Hands-on Practice
Modify the program to find all prime numbers within a given range.
Optimize further using the Sieve of Eratosthenes.
11. Problem 9: Reversing a Number
Why This Problem?
Reversing a number is a common problem that helps in understanding mathematical operations, loops, and string manipulation. It is often used in palindromes and encryption algorithms.
Solution and Explanation
Method 1: Using a Loop
We extract each digit one by one using the modulus and division operations.
num = int(input(“Enter a number: “))
rev = 0
while num > 0:
remainder = num % 10
rev = (rev * 10) + remainder
print(“Reversed number:”, rev)
Method 2: Using String Slicing
Python allows reversing strings easily using slicing:
num = input(“Enter a number: “)
print(“Reversed number:”, num[::-1])
Edge Cases
How does the program handle negative numbers?
What happens if the number has trailing zeros (e.g., 1200 → 21)?
Hands-on Practice
Modify the program to check if the reversed number is equal to the original (palindrome check).
12. Problem 10: Checking for Palindromes
Why This Problem?
Palindrome numbers and strings read the same forward and backward. This problem strengthens string manipulation and comparison skills.
Solution and Explanation
Method 1: Using String Comparison
python
num = input(“Enter a number: “)
if num == num[::-1]:
print(num, “is a palindrome.”)
Method 2: Using a Loop
We reverse the number manually and check if it matches the original.
python
num = int(input(“Enter a number: “))
original = num
rev = 0
while num > 0:
rev = rev * 10 + (num % 10)
num //= 10
if original == rev:
print(original, “is a palindrome.”)
Edge Cases
Palindrome check for strings (“madam”, “racecar”)
Handling spaces and case sensitivity (“No lemon, no melon”)
Hands-on Practice
Extend the program to check for palindromic words while ignoring case and spaces.
13. Problem 11: Finding the Sum of Digits of a Number
Why This Problem?
Summing digits is a fundamental operation used in checksum algorithms, number theory, and cryptography.
Solution and Explanation
Method 1: Using a Loop
We extract digits one by one using modulus (%) and division (//).
python
num = int(input(“Enter a number: “))
sum_digits = 0
while num > 0:
sum_digits += num % 10
num //= 10
print(“Sum of digits:”, sum_digits)
Method 2: Using Recursion
python
def sum_of_digits(n):
if n == 0:
return 0
return (n % 10) + sum_of_digits(n // 10)
num = int(input(“Enter a number: “))
print(“Sum of digits:”, sum_of_digits(num))
Edge Cases
Handling negative numbers
Checking single-digit numbers
Hands-on Practice
Modify the program to keep summing digits until a single-digit number is reached.
14. Problem 12: Counting Vowels and Consonants in a String
Why This Problem?
This problem is useful for text processing, NLP (natural language processing), and building search algorithms.
Solution and Explanation
python
string = input(“Enter a string: “).lower()
vowels = “aeiou”
vowel_count = consonant_count = 0
for char in string:
if char.isalpha():
if char in vowels:
vowel_count += 1
else:
consonant_count += 1
print(“Vowels:”, vowel_count)
print(“Consonants:”, consonant_count)
Understanding the Logic
Convert the string to lowercase to handle case sensitivity.
Use isalpha() to ignore spaces and special characters.
Edge Cases
Handling spaces, numbers, and special characters
Testing with uppercase and lowercase letters
Hands-on Practice
Modify the program to count digits and special characters separately.
15. Problem 13: Finding the Length of a String Without len()
Why This Problem?
While Python has built-in functions like len(), learning to calculate the length manually helps understand loops and iteration.
Solution and Explanation
python
string = input(“Enter a string: “)
count = 0
for char in string:
count += 1
print(“Length of string:”, count)
Using a While Loop
python
string = input(“Enter a string: “)
index = 0
while True:
try:
string[index]
index += 1
except IndexError:
break
print(“Length of string:”, index)
Edge Cases
What happens if the string is empty?
How does it handle spaces and special characters?
Hands-on Practice
Modify the program to calculate the length of a list or tuple without using len().
16. Problem 14: Removing Duplicates from a List
Why This Problem?
This problem is important when working with data structures, as duplicate removal is common in databases, search algorithms, and machine learning preprocessing.
Solution and Explanation
Method 1: Using a Loop
python
numbers = [1, 2, 2, 3, 4, 4, 5, 6, 6]
unique_numbers = []
for num in numbers:
if num not in unique_numbers:
unique_numbers.append(num)
print(“List without duplicates:”, unique_numbers)
Method 2: Using a Set
Python sets automatically remove duplicates.
python
numbers = [1, 2, 2, 3, 4, 4, 5, 6, 6]
unique_numbers = list(set(numbers))
print(“List without duplicates:”, unique_numbers)
Preserving Order
Sets don’t maintain order, so we can use dict.fromkeys():
python
numbers = [1, 2, 2, 3, 4, 4, 5, 6, 6]
unique_numbers = list(dict.fromkeys(numbers))
print(“List without duplicates:”, unique_numbers)
Hands-on Practice
Modify the program to remove duplicates from a string while preserving order.
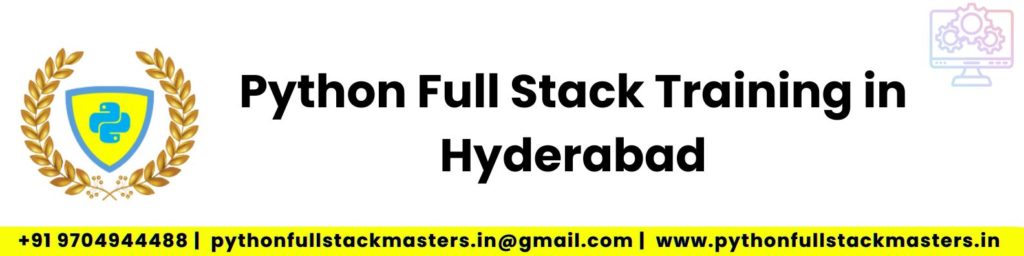
17. Problem 15: Checking for Anagrams
Why This Problem?
Anagrams are widely used in text processing, encryption, and language processing applications.
Solution and Explanation
An anagram is when two words contain the same letters in a different order (e.g., “listen” and “silent”).
Method 1: Sorting Approach
python
def is_anagram(str1, str2):
return sorted(str1) == sorted(str2)
word1 = input(“Enter first word: “)
word2 = input(“Enter second word: “)
if is_anagram(word1.lower(), word2.lower()):
print(“The words are anagrams.”)
else:
print(“The words are not anagrams.”)
Method 2: Using a Dictionary (More Efficient)
python
from collections import Counter
def is_anagram(str1, str2):
return Counter(str1) == Counter(str2)
word1 = input(“Enter first word: “)
word2 = input(“Enter second word: “)
print(“Anagram:”, is_anagram(word1.lower(), word2.lower()))
Hands-on Practice
Modify the program to ignore spaces and punctuation when checking for anagrams.
18. Problem 16: Finding the Second Largest Number in a List
Why This Problem?
This is a common problem in coding interviews and helps in understanding sorting and conditional logic.
Solution and Explanation
Method 1: Sorting Approach
python
numbers = [12, 35, 1, 10, 34, 1]
numbers = list(set(numbers)) # Remove duplicates
numbers.sort()
print(“Second largest number:”, numbers[-2])
Method 2: Without Sorting (Efficient Approach)
python
numbers = [12, 35, 1, 10, 34, 1]
first, second = float(‘-inf’), float(‘-inf’)
for num in numbers:
if num > first:
second, first = first, num
elif num > second and num != first:
second = num
print(“Second largest number:”, second)
Hands-on Practice
Modify the program to find the second smallest number in the list.
19. Problem 17: Merging Two Sorted Lists
Why This Problem?
Merging sorted lists is fundamental in sorting algorithms like Merge Sort and is commonly used in database operations.
Solution and Explanation
Method 1: Using sorted()
python
list1 = [1, 3, 5, 7]
list2 = [2, 4, 6, 8]
merged_list = sorted(list1 + list2)
print(“Merged sorted list:”, merged_list)
Method 2: Using Two-Pointer Approach (More Efficient)
python
list1 = [1, 3, 5, 7]
list2 = [2, 4, 6, 8]
merged_list = []
i, j = 0, 0
while i < len(list1) and j < len(list2):
if list1[i] < list2[j]:
<mark id=”p_42″>merged_list.append(list1[i])
i += 1
else:
merged_list.append(list2[j])
j += 1
merged_list.extend(list1[i:])
merged_list.extend(list2[j:])
print(“Merged sorted list:”, merged_list)
20. Conclusion
We’ve covered a variety of Python practice problems that introduce you to key concepts such as:
Basic operations: Printing, arithmetic, swapping
Logical conditions: Even/odd, largest number, prime numbers
Loops and recursion: Factorials, Fibonacci, sum of digits
String and list operations: Palindromes, anagrams, merging lists
Why Practice Is Important?
Solving coding problems helps you build problem-solving skills and prepare for interviews and real-world programming challenges.
What’s Next?
Now that you have completed these beginner-level problems, you can:
Try intermediate challenges like sorting algorithms, recursion problems, and object-oriented programming.
Explore platforms like LeetCode, CodeWars, and HackerRank for more practice.
Work on small projects like a to-do list app, calculator, or text-based game.
FAQS
1. Who is this for?
This is for beginners who want to practice Python and build confidence in coding. No prior experience is needed
2. What topics do these problems cover?
These problems cover basic Python concepts like variables, loops, conditionals, functions, lists, and more.
3. Do I need any special software to solve these problems?
No, you only need Python installed on your computer or you can use an online Python compiler.
4. How difficult are these problems?
They are beginner-friendly, starting from simple exercises and gradually increasing in difficulty.
5. Are there solutions available?
Yes! Many problems include solutions and explanations to help you learn effectively.
6. Can I use these problems to prepare for coding interviews?
Yes! While they are beginner-focused, they help build problem-solving skills useful for technical interviews.
7. How can I get better at solving Python problems?
Practice consistently, read explanations, and try modifying problems to explore different solutions.
8. Where can I practice these problems?
You can use online platforms like LeetCode, HackerRank, or free Python coding
9. Do I need to know advanced math for these problems?
No, most problems require only basic arithmetic and logical thinking.
10. Can I use these problems for self-study or in a classroom?
Yes! These problems are great for self-learning and can be used by teachers for classroom exercises.
The Python Full Stack Training is a 2 months program offering online and offline classes. We covers Python fundamentals, front-end technologies like HTML training, CSS training, and React training and back-end frameworks such as Django and Flask. With hands-on project , expert guidance and free batch access until get placement."