Python Full Stack Syllabus
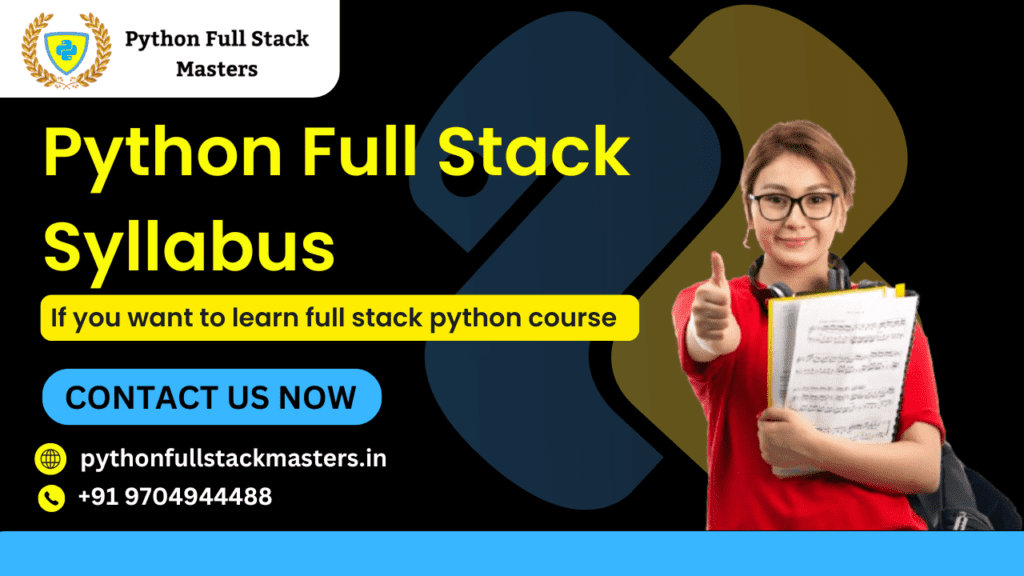
Overview of Python Full Stack Syllabus
- If you want to start your career as a python full stack developer initially you have to know the syllabus and prepare a plan to learn.
- Here in this blog we explained the syllabus of python full stack development course that will help you easily understand and easy to follow.
- The Python Full Stack syllabus is a well structured guide to learning how to build complete web applications using Python.
- It covers everything you need to know, from the basics of Python programming to building both the front and back ends of a website, and even adding artificial intelligence (AI) features.
Python full stack syllabus includes:
- Python Basics: Learn the core Python programming language, including variables, loops, functions, and object-oriented programming.
- Backend Development: Understand how to create the server-side of your web application using Python frameworks like Flask or Django. You’ll also learn how to connect your app to databases to store data.
- Frontend Development: Discover how to create the user-facing part of your web app with HTML, CSS, and JavaScript. Learn to make your site interactive using frontend frameworks like React.js.
- Integrating AI: Learn how to add AI capabilities to your app, such as chatbots or recommendation systems, using Python’s AI libraries like TensorFlow or scikit-learn.
- Building Real Projects: Put your knowledge into practice by creating AI-powered web applications that you can deploy and share with the world.
- Deployment and Optimization: Learn how to deploy your app to the cloud, handle real traffic, and optimize performance.
- Python Full Stack Development, including the basics like setting up the Python environment and a detailed breakdown of the Python syllabus with relevant subtopics.
- This will help users get started from the very beginning, leading them through Python programming and full-stack web development concepts.
Python Full Stack Course Syllabus: A Complete Guide
- Python Full Stack Development offers a deep understanding of both the front-end and back-end aspects of web development, with Python as the central programming language.
- Whether you’re just starting with Python or you’re ready to dive into full-stack development, this guide will break down each topic step-by-step. Let’s start from the very basics!
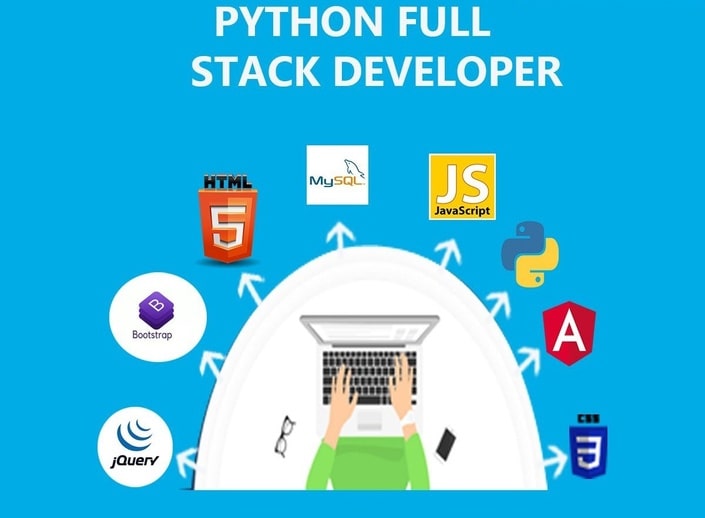
1. Python Basics and Setting Up the Development Environment
- Before you start full-stack development, it’s very important to understand Python programming and how to set up your environment.
Setting Up Python Environment:
- Setting up a proper Python environment is the first step toward your development journey. The environment allows you to install libraries, manage versions, and run your Python scripts.
- Installing Python: Download and install Python from python.org.
- Using pip: Learn how to install packages using pip (Python package manager).
- Setting up a Virtual Environment:
- Why use a virtual environment?
- A virtual environment is used to isolate a project’s dependencies, ensuring that each project has its own set of packages and their specific versions, preventing conflicts between different projects on the same system, thereby promoting reproducibility and reliable execution of your code across different environments
- Creating a virtual environment using venv or virtualenv.
- Activating and deactivating virtual environments.
- Installing IDE: Install an Integrated Development Environment (IDE) like VS Code, PyCharm, or Sublime Text.
- Jupyter Notebooks: For data science or testing small scripts, installing Jupyter Notebook.
Python Syllabus and Core Topics
- Once the environment is ready, let’s explore the Python language and its fundamentals.
- Introduction to Python:
- Python syntax, indentation, and coding conventions.
- Differences between Python 2.x and Python 3.x.
- Variables and Data Types:
- Understanding variables, constants, and different data types (int, float, string, boolean, etc.).
- Type conversion: int(), float(), str().
- Control Structures:
- Conditionals: if, elif, else statements.
- Loops: for, while loops and range().
- Functions:
- Defining functions using def.
- Parameters, return values, and scope.
- Lambda functions (anonymous functions).
- Data Structures:
- Lists: Accessing, modifying, slicing, and methods like append(), remove(), sort().
- Tuples: Creating immutable sequences.
- Dictionaries: Key-value pairs and dictionary methods (keys(), values(), items()).
- Sets: Unique collections and set operations (union, intersection).
- Object-Oriented Programming (OOP):
- Classes and Objects: Creating classes and objects.
- Attributes and Methods: Instance variables and class methods.
- Inheritance: Understanding parent-child class relationships.
- Polymorphism and Encapsulation: Function overloading, method overriding.
- Error Handling and Exceptions:
- Try-Except: Handling errors with try, except, and finally.
- Custom error handling using raise and creating custom exceptions.
- File Handling:
- Reading and Writing Files: Using open(), read(), write(), and with statements for context management.
2. Web Development with Python: Back-End Technologies
Web Frameworks: Flask vs Django:
- Python offers two popular frameworks for back-end web development. One is Flask (lightweight) and another is Django (full-stack). You can choose one depending on your project’s needs.
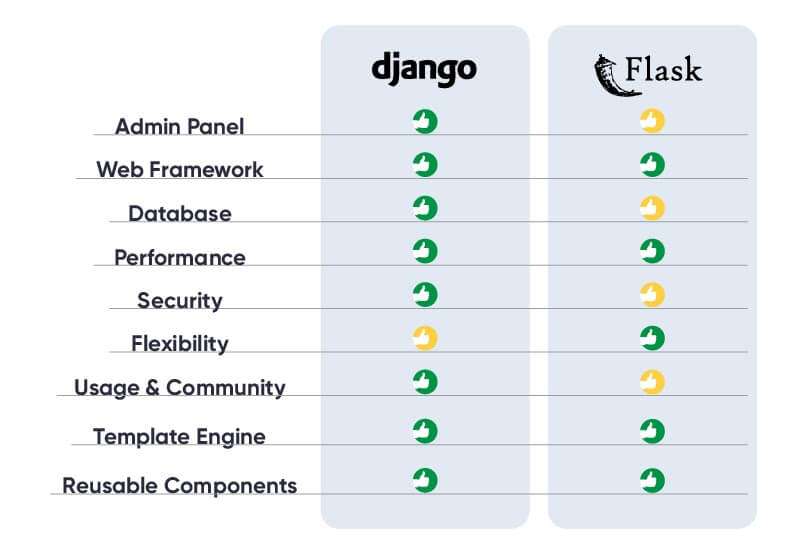
1.Flask Framework (Micro-framework):
- Flask is a minimalistic framework for small to medium web applications. It gives you more control over the components of your application.
- Setting up Flask: Install Flask using pip install flask.
- Flask App Structure: Creating the basic structure of a Flask app, including routes and templates.
- Routing: Using @app.route() to define URL paths.
- Request and Response: Understanding HTTP methods (GET, POST) and interacting with request data.
- Template Rendering with Jinja2: Dynamically rendering HTML templates.
- Form Handling: Handling user input from HTML forms.
- Flask Extensions: Using extensions like Flask-SQLAlchemy for database management and Flask-Login for user authentication.
2.Django Framework (Full-stack framework):
- Django is a more feature-rich framework designed for larger applications with built-in tools for user authentication, database management, and admin interfaces.
- Setting up Django: Install Django using pip install django and create a new Django project using django-admin startproject.
- Django App Structure: Understanding the directory structure and settings in a Django project.
- URL Routing: Mapping URLs to views with Django’s URL dispatcher.
- Models and ORM: Understanding Django’s ORM for interacting with relational databases.
- Admin Interface: Using the Django admin panel to manage content.
- Django Templates: Rendering dynamic content using Django’s template language.
- User Authentication: Handling user login, registration, and permissions.
Database Technologies (SQL & NoSQL):
- Databases store and manage data for your web application. You’ll need to learn both relational databases (SQL) and non-relational databases (NoSQL).
1.SQL Databases (PostgreSQL/MySQL):
- SQL Queries: Writing SELECT, INSERT, UPDATE, DELETE queries.
- Joins: Combining data from multiple tables using INNER JOIN, LEFT JOIN.
- Relationships: One-to-one, one-to-many, and many-to-many relationships.
- Database Design: Normalization and indexing for optimized performance.
2.NoSQL Databases (MongoDB):
- Document-Based Storage: Understanding collections and documents in MongoDB.
- CRUD Operations: Performing Create, Read, Update, Delete operations in MongoDB.
- Aggregation: Using the aggregation framework for complex data queries.
Building RESTful APIs:
- APIs (Application Programming Interfaces) allow your back-end to communicate with front-end applications or other services.
- What is REST?:
- Understanding the principles of REST (Representational State Transfer).
- Creating APIs with Flask/Django:
- Building RESTful APIs using Flask and Django REST Framework (DRF).
- Serializing data to JSON format.
- Setting up routes for API endpoints and handling GET, POST, PUT, DELETE requests.
- Authentication and Authorization:
- JWT (JSON Web Tokens): Secure API communication by generating and verifying tokens.
- OAuth: Integrating third-party logins (Google, Facebook).
3. Front-End Technologies (Client-Side):
- As a full-stack developer, you must also understand the technologies that power the user interface of a web application.
HTML (HyperText Markup Language):
- HTML provides the structure for web pages by defining headings, paragraphs, lists, links, images, forms, and other elements.
- Basic Structure: Understanding the HTML document structure (html, head, body).
- Text Formatting: Using headings (<h1>, <h2>), paragraphs (<p>), and text styles (<b>, <i>, <u>).
- Links: Creating hyperlinks using <a> tags.
- Forms: Handling user input with <form>, <input>, <select>, <textarea>, and <button> tags.
CSS (Cascading Style Sheets):
- CSS is used to style the HTML elements, controlling the layout, design, and responsiveness of a web page.
- CSS Selectors: Understanding the different types of selectors (class, ID, element).
- Box Model: Understanding padding, margin, border, and content.
- Flexbox & Grid: Modern layout techniques for creating responsive designs.
- Responsive Design: Making pages mobile-friendly using media queries.
- Animations and Transitions: Adding dynamic visual effects to your pages.
JavaScript:
- JavaScript adds interactivity and dynamic behavior to web pages.
- Variables and Data Types: Using let, const, var, strings, arrays, and objects.
- Functions and Control Flow: Writing functions, handling conditions with if-else, and looping.
- DOM Manipulation: Interacting with HTML elements using JavaScript.
- Events: Responding to user interactions (clicks, keystrokes) with event listeners.
- Async Programming: Using Promises, async/await, and handling API requests asynchronously.
4. Full Stack Development Integration:
- After mastering both front-end and back-end, it’s time to integrate the two.
Connecting Front-End with Back-End
- Now you’ll learn how to connect the user interface (front-end) with the server (back-end).
- Making API Calls from Front-End: Using JavaScript’s fetch() API to get and display data from the server.
- Handling User Input: Submitting forms and capturing user input via HTTP requests.
- Authentication: Handling user sessions and securely storing authentication tokens.
5. Advanced Topics
- These topics will help you take your full-stack development skills to the next level.
Cloud Deployment and DevOps:
- Deploy your application to the cloud for production.
- Deployment on Heroku: Deploying Flask or Django applications to Heroku.
- AWS or Google Cloud: Using cloud platforms to host your application and manage resources.
- CI/CD: Setting up Continuous Integration and Continuous Deployment pipelines.
Machine Learning and AI:
- Integrate machine learning and artificial intelligence into your applications.
- Using Python Libraries: Incorporating machine learning models with libraries like TensorFlow, Keras, or scikit-learn.
- Integrating AI in Web Apps: Deploying models as APIs for intelligent recommendations or predictions.
Why to choose python over other programming languages:
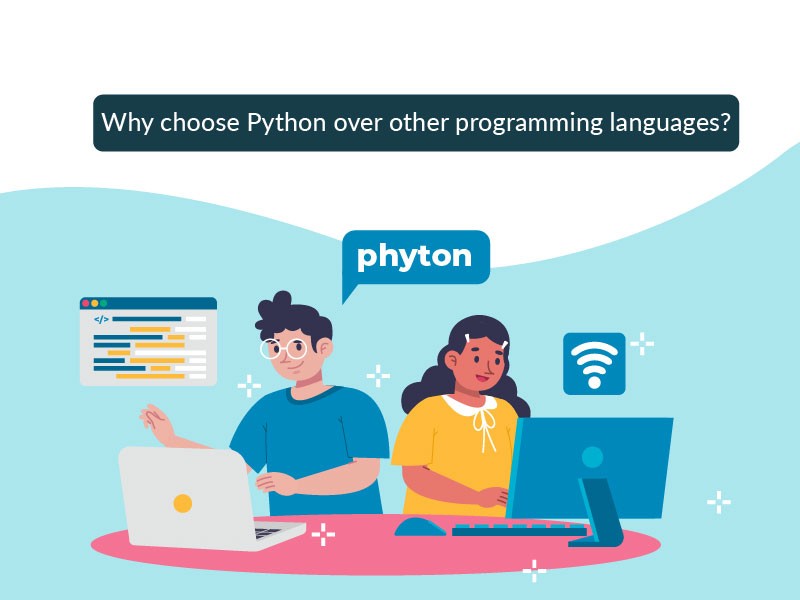
1. Easy to Learn:
2. Super Versatile:
- You can do a lot with Python. Whether you want to build websites, analyze data, make games, automate tasks, or create smart systems like AI, Python can handle it.
- That’s why it’s so popular across different fields.
3. Big Support Community:
- Python has a huge community of developers. That means if you run into a problem, you can find lots of help, tutorials, and pre-built code to use (called libraries) to make your work faster.
- This is super helpful when you’re just starting out or need help on a tough problem.
4. Works Everywhere:
5. Free to Use:
- Python is open-source, meaning it’s completely free to download and use. You don’t have to pay for anything, and you can even modify it if you need to.
6. Easy to Integrate with Other Technologies:
- You can use Python alongside other programming languages and technologies. For example, if you’re building a website, Python can work with other web technologies, or if you need to work with a database, Python can connect to it easily.
7. Quick to Build Things:
- Python lets you write code faster. If you want to quickly test an idea or create a simple version of a product (called a prototype), Python helps you get there in less time compared to many other languages.
8. Good for Working with Data and AI:
- Python is the go-to language for data analysis, AI (like robots or smart assistants), and machine learning. It has great tools (called libraries) that make working with data easier and faster, which is why it’s popular in fields like science, tech, and finance.
9. Perfect for Automating Tasks:
- If you find yourself doing repetitive computer tasks (like renaming files or checking for updates), Python is great for automating those tasks, saving you time and effort.
10. Great Documentation:
- The official Python documentation is really well-written, and it’s easy to find answers to your questions. You can learn how to use the tools and libraries in Python without too much confusion.
11. Used by Big Companies:
12. Quick and Easy to Understand Code:
- Python code is very readable. Once you write it, even other people can look at it and quickly understand what it does. This is important when you’re working in teams or revisiting your code after some time.
Additional resources:
Conclusion
- Becoming proficient in Python Full Stack Development requires learning and mastering both front-end and back-end technologies.
- This syllabus guides you through Python programming, web development frameworks (Flask, Django), front-end skills (HTML, CSS, JavaScript), and more.
- Starting from the basics of Python, you will progress to complex topics like machine learning and cloud deployment, allowing you to create powerful, scalable, and intelligent web applications.
The Python Full Stack Training is a 2 months program offering online and offline classes. We covers Python fundamentals, front-end technologies like HTML training, CSS training, and React training and back-end frameworks such as Django and Flask. With hands-on project , expert guidance and free batch access until get placement."
{ Near JNTU Metro Station,3rd Floor,Atmaram Estates,} {beside TATA Motors,Hyder Nagar,}, {Hyderabad, Telangana} {500072}
Monday, Tuesday, Wednesday, Thursday, Friday, Saturday8:00 am – 8:00 pm
Python Fullstack Masters
20