Python Beginner Exercises
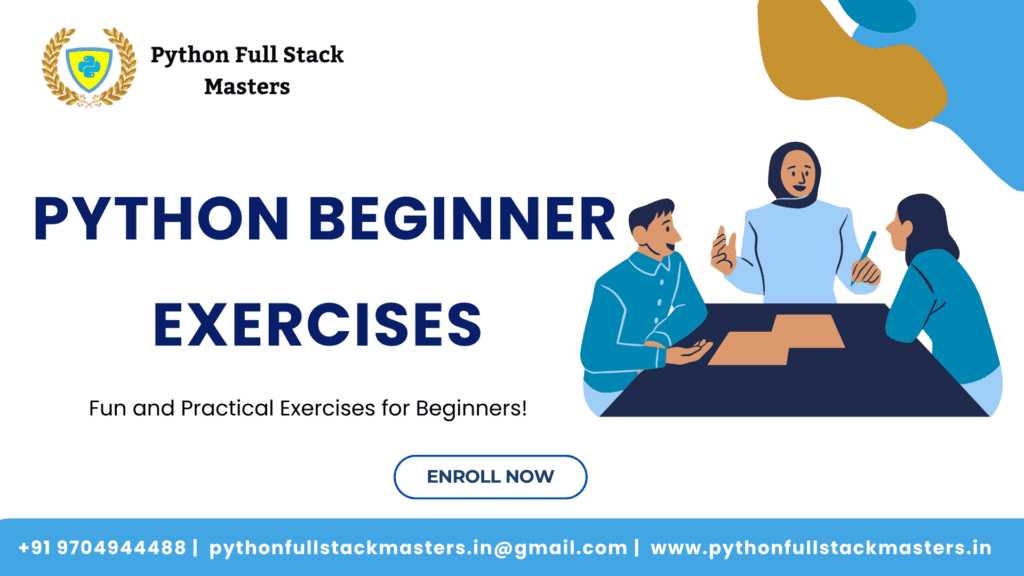
1. Introduction to Python Beginners Exercises
Python is one of the most beginner-friendly programming languages, making it the perfect choice for those new to coding. It is known for its simple and readable syntax, which closely resembles natural language. Python is used in a variety of fields, including web development, data science, artificial intelligence, automation, and more.
One of the reasons Python is so popular is that it does not require complex syntax like some other programming languages. Unlike Java or C++, Python does not need semicolons or curly brackets to structure the code. This simplicity makes it easier for beginners to focus on learning logic and problem-solving rather than struggling with syntax.
Another advantage of Python is its vast ecosystem of libraries and frameworks. Whether you are interested in data science (NumPy, Pandas), web development (Flask, Django), or automation (Selenium), Python has a library for nearly every purpose.
By learning Python, you open the door to a vast world of opportunities in technology and software development. The exercises in this article will guide you from basic concepts to more advanced topics, helping you build a strong foundation in Python programming.
2. Setting Up Your Python Environment
This involves installing Python on your computer and choosing an Integrated Development Environment (IDE) to write and execute your code.
Installing Python
Python is available for Windows, macOS, and Linux. Follow these steps to install Python:
Once installed, open a terminal or command prompt and type python –version to confirm the installation.
Choosing an IDE
An IDE (Integrated Development Environment) is a tool that makes coding easier by providing features like syntax highlighting, debugging, and code suggestions. Some popular IDEs for Python include:
IDLE (comes pre-installed with Python)
VS Code (lightweight and highly customizable)
PyCharm (a powerful IDE for professional developers)
For beginners, IDLE or VS Code is a good choice.
3. Understanding Python Syntax and Basic Concepts
Python has a clean and readable syntax. Unlike other languages, it does not use semicolons (;) to mark the end of a statement. Instead, Python relies on indentation (whitespace) to define code blocks.
Python Indentation
Indentation is crucial in Python. Instead of using {} (curly brackets) like C or Java, Python uses indentation to define blocks of code. Example:
python
if 5 > 2:
print(“Five is greater than two”)
If you forget to indent properly, Python will throw an IndentationError.
Variables and Data Types
Variables in Python do not need explicit declaration. The interpreter assigns the data type dynamically.
python
name = “Alice” # String
age = 25 # Integer
height = 5.6 # Float
is_student = True # Boolean
Python also supports complex data types like lists, tuples, and dictionaries, which we will explore later.
4. Exercise 1: Printing "Hello, World!"
Every beginner starts with the classic “Hello, World!” program. In Python, it’s as simple as:
python
print(“Hello, World!”)
Why is This Important?
It helps you verify that Python is installed correctly.
It introduces the print() function, which is essential for outputting text.
It builds confidence as you run your first successful script.
To execute this program:
Open your Python IDE.
Type print(“Hello, World!”) and save it as hello.py.
Run the script using the command python hello.py.
You should see Hello, World! printed on the screen!
5. Exercise 2: Basic Arithmetic Operations
Python can perform basic arithmetic like addition, subtraction, multiplication, and division.
python
a = 10
b = 5
print(“Addition:”, a + b)
print(“Subtraction:”, a – b)
print(“Multiplication:”, a * b)
print(“Division:”, a / b)
Operators in Python
Operator
Description
Example
+
Addition
5 + 3 = 8
–
Subtraction
5 – 3 = 2
*
Multiplication
5 * 3 = 15
/
Division
5 / 2 = 2.5
Python also supports other mathematical functions like exponentiation (**), modulus (%), and floor division (//).
6. Exercise 3: Variables and User Input
One of the fundamental concepts in programming is handling user input and storing values in variables. Variables store information that can be used and modified later.
Declaring Variables
In Python, you don’t need to specify the data type of a variable explicitly.python
name = “Alice” # String
age = 25 # Integer
height = 5.5 # Float
is_student = True # Boolean
Python is dynamically typed, meaning the type of a variable can change during execution.
python
x = 10 # x is an integer
x = “Hello” # Now x is a string
Taking User Input
The input() function allows the user to enter values during program execution. By default, input() returns a string.
name = input(“Enter your name: “)
print(“Hello, ” + name + “!”)
If you want to take numerical input, you must convert it using int() or float().
python
age = int(input(“Enter your age: “))
print(“Next year, you will be”, age + 1)
Why is This Exercise Important?
It introduces the concept of user interaction.
It teaches variable declaration and data types.
It builds a foundation for creating interactive programs.
7. Exercise 4: Conditional Statements (if, elif, else)
Conditional statements allow your program to make decisions based on conditions. Python provides if, elif, and else statements.
Basic If-Else Example
python
age = int(input(“Enter your age: “))
if age >= 18:
print(“You are eligible to vote.”)
else:
print(“You are not eligible to vote.”)
Using elif for Multiple Conditions
python
marks = int(input(“Enter your marks: “))
if marks >= 90:
print(“Grade: A”)
elif marks >= 80:
print(“Grade: B”)
elif marks >= 70:
print(“Grade: C”)
else:
print(“Grade: F”)
Comparison and Logical Operators in Conditions
Operator
Description
Example
==
Equal to
a == b
!=
Not equal to
a != b
>
Greater than
a > b
<
Less than
a < b
>=
Greater than or equal to
a >= b
<=
Less than or equal to
a <= b
and
Both conditions must be true
a > 5 and a < 10
or
At least one condition must be true
a > 5 or a < 3
not
Negates a condition
not(a > 5)
Why is This Exercise Important?
It introduces decision-making in programming.
It helps understand logical operators.
It is essential for writing dynamic and interactive applications.
8. Exercise 5: Loops in Python (for and while loops)
For Loop Example
A for loop iterates over a sequence like a list or a string.
python
for i in range(5): # 0 to 4
print(“Iteration:”, i)
While Loop Example
A while loop runs as long as a condition remains true.
python
count = 0
while count < 5:
print(“Count:”, count)
count += 1
Loop Control Statements
break – Exits the loop.
<mark id=”p_10″>continue – Skips the current iteration and moves to the next one.
python
for i in range(10):
if i == 5:
break # Stops the loop at 5
print(i)
for i in range(10):
if i == 5:
continue # Skips 5
print(i)
Why is This Exercise Important?
Loops are essential for automation.
They help reduce code duplication.
They improve efficiency by handling repetitive tasks.
9. Exercise 6: Functions and Their Importance
Functions help break a program into smaller, reusable blocks of code.
Defining a Function
python
def greet():
print(“Hello, welcome to Python!”)
Calling a Function
python
greet() # Outputs: Hello, welcome to Python!
Function with Parameters
python
def greet(name):
print(“Hello,”, name)
greet(“Alice”)
Returning Values from a Function
python
def square(num):
return num * num
result = square(5)
print(“Square of 5:”, result)
Why is This Exercise Important?
Functions promote code reuse.
They improve readability and maintainability.
They allow modular programming.
10. Exercise 7: Working with Lists and Tuples
Lists and tuples store multiple values in a single variable.
Lists (Mutable)
Lists are ordered and changeable.
python
fruits = [“apple”, “banana”, “cherry”]
fruits.append(“orange”) # Adds a new element
print(fruits) # [‘apple’, ‘banana’, ‘cherry’, ‘orange’]
Tuples (Immutable)
Tuples are like lists but cannot be changed after creation.
python
colors = (“red”, “green”, “blue”)
print(colors[0]) # Outputs: red
List Operations
Operation
Example
Append
list.append(item)
Remove
list.remove(item)
Sort
list.sort()
Reverse
list.reverse()
Tuple Operations
Operation
Example
Accessing elements
tuple[index]
Length
len(tuple)
Why is This Exercise Important?
Lists and tuples are fundamental in Python.
They are used for data storage and manipulation.
Understanding them helps in handling collections of data.
11. Exercise 8: Working with Dictionaries in Python
Dictionaries in Python store data as key-value pairs, making them useful for structured data representation. Unlike lists, where data is indexed by position, dictionaries use unique keys to access values.
Creating a Dictionary
python
student = {
“name”: “Alice”,
“age”: 21,
“grade”: “A”
}
print(student[“name”]) # Outputs: Alice
Adding and Updating Dictionary Values
python
student[“age”] = 22 # Update age
student[“subject”] = “Math” # Add a new key-value pair
print(student)
Removing Elements from a Dictionary
python
del student[“grade”] # Removes “grade” key
print(student)
Looping Through a Dictionary
python
for key, value in student.items():
print(key, “:”, value)
Why is This Exercise Important?
Dictionaries allow efficient data retrieval.
They are commonly used in APIs and real-world applications.
They help in organizing and structuring data logically.
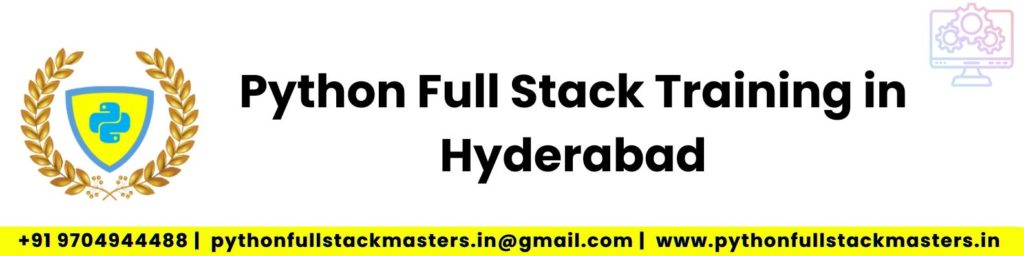
12. Exercise 9: String Manipulation
Strings in Python are sequences of characters, and Python provides many built-in methods for string manipulation.
String Indexing and Slicing
python
text = “Python Programming”
print(text[0]) # Outputs: P
print(text[-1]) # Outputs: g
print(text[0:6]) # Outputs: Python
Common String Methods
Method
Description
Example
lower()
Converts to lowercase
“HELLO”.lower() → “hello”
upper()
Converts to uppercase
“hello”.upper() → “HELLO”
strip()
Removes whitespace
” hello “.strip() → “hello”
replace()
Replaces a substring
“Hello”.replace(“H”, “J”) → “Jello”
split()
Splits a string into a list
“a,b,c”.split(“,”) → [“a”, “b”, “c”]
Why is This Exercise Important?
String manipulation is essential in text processing.
It helps in data cleaning and formatting.
It is widely used in web scraping and automation.
13. Exercise 10: List Comprehension
List comprehension offers a concise way to create lists based on existing lists or other iterable objects.
Basic List Comprehension
python
numbers = [x for x in range(10)]
print(numbers) # Outputs: [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
Using Functions in List Comprehension
python
def square(num):
return num * num
Why is This Exercise Important?
It makes code more readable and efficient.
It is useful for filtering and transforming data.
It is widely used in data science and machine learning.
14. Exercise 11: File Handling in Python
Python allows reading from and writing to files, making it useful for working with external data.
Writing to a File
python
with open(“sample.txt”, “w”) as file:
file.write(“Hello, this is a sample file.”)
Reading from a File
python
with open(“sample.txt”, “r”) as file:
content = file.read()
print(content)
Appending to a File
python
with open(“sample.txt”, “a”) as file:
file.write(“\nThis is a new line.”)
Why is This Exercise Important?
It enables saving and retrieving user-generated data.
It is widely used in automation and data logging.
15. Exercise 12: Exception Handling (Try, Except, Finally)
Handling errors in Python ensures a smooth user experience and prevents program crashes.
Basic Try-Except Block
python
try:
x = 10 / 0 # This will cause a ZeroDivisionError
except ZeroDivisionError:
print(“Error: Cannot divide by zero!“)
Handling Multiple Exceptions
python
try:
num = int(input(“Enter a number: “))
print(10 / num)
except ZeroDivisionError:
print(“Cannot divide by zero!”)
except ValueError:
print(“Invalid input! Please enter a number.”)
Finally Block
python
try:
file = open(“data.txt”, “r”)
print(file.read())
except FileNotFoundError:
print(“File not found!”)
finally:
print(“Execution completed.”)
Why is This Exercise Important?
Prevents crashes due to unexpected errors.
Enhances user experience by handling exceptions gracefully.
Improves code reliability.
16. Exercise 13: Object-Oriented Programming (OOP) Basics
OOP allows structuring programs around objects and classes.
Creating a Class and Object
python
class Car:
def __init__(self, brand, model):
self.brand = brand
self.model = model
def display_info(self):
print(f”Car: {self.brand} {self.model}”)
car1 = Car(“Toyota”, “Camry”)
car1.display_info() # Outputs: Car: Toyota Camry
Encapsulation, Inheritance, and Polymorphism
python
class ElectricCar(Car):
def __init__(self, brand, model, battery_capacity):
super().__init__(brand, model)
self.battery_capacity = battery_capacity
def display_info(self):
print(f”Electric Car: {self.brand} {self.model}, Battery: {self.battery_capacity} kWh”)
car2 = ElectricCar(“Tesla”, “Model S”, 100)
car2.display_info() # Outputs: Electric Car: Tesla Model S, Battery: 100 kWh
Why is This Exercise Important?
OOP improves code organization and reusability.
It is widely used in software development.
It makes large projects more manageable.
17. Exercise 14: Working with Modules and Importing Libraries
Python provides built-in and external modules that allow developers to extend the functionality of their programs. A module is simply a file containing Python code, such as functions and classes, that can be imported and used in other scripts.
Importing Built-in Modules
Python comes with a variety of built-in modules that you can use without installing anything.
python
import math
print(math.sqrt(25)) # Outputs: 5.0
print(math.factorial(5)) # Outputs: 120
Creating and Importing Custom Modules
You can also create your own module and import it into another script.
Create a file mymodule.py:
python
def greet(name):
return f”Hello, {name}!”
Import and use it in another script:
python
import mymodule
print(mymodule.greet(“Alice”))
Installing and Using External Modules
You can install third-party modules using pip.
pip install requests
Then, use the installed module:
python
import requests
response = requests.get(“https://www.google.com”)
print(response.status_code)
Why is This Exercise Important?
Modules promote code reusability.
They enhance Python’s functionality.
Many real-world applications rely on external libraries.
18. Exercise 15: Basic Data Analysis with Pandas
Installing Pandas
pip install pandas
Reading and Displaying a CSV File
Python
import pandas as pd
df = pd.read_csv(“data.csv”)
print(df.head()) # Displays the first 5 rows
Why is This Exercise Important?
Pandas is essential for data science and analytics.
It makes handling large datasets easy.
It is widely used in machine learning.
19. Exercise 16: Introduction to NumPy for Beginners
Installing NumPy
sh
pip install numpy
Creating NumPy Arrays
python
import numpy as np
arr = np.array([1, 2, 3, 4, 5])
print(arr)
Performing Mathematical Operations
python
print(arr * 2) # Multiplies each element by 2
print(np.mean(arr)) # Calculates the mean
Why is This Exercise Important?
NumPy is essential for numerical computing.
It is widely used in data science and AI.
It optimizes performance compared to Python lists
20. Conclusion and Next Steps in Python
Congratulations on completing these Python beginner exercises! By now, you should have a strong understanding of Python’s core concepts.
Key Takeaways:
Python syntax is simple and easy to learn.
Variables, loops, and conditionals form the foundation of programming.
Lists, dictionaries, and functions improve code organization.
Working with files and modules enhances program functionality.
Libraries like Pandas and NumPy are powerful for data analysis.
What’s Next?
Explore Object-Oriented Programming (OOP) in more depth.
Learn about APIs and how to interact with web services.
Dive into data science and machine learning with Python.
Start building real-world projects like a to-do app or a weather bot.
FAQS
Is Python good for beginners?
Yes! Python’s simple syntax and readability make it perfect for beginners.
What is the best way to practice Python?
Work on small projects, solve coding problems, and build real-world applications.
Do I need a strong math background to learn Python?
No, but basic logic and problem-solving skills are helpful.
Can I use Python for web development?
Yes! Frameworks like Django and Flask are great for web development.
What are some beginner-friendly Python projects?
Try building a calculator, to-do list, or simple game like Tic-Tac-Toe.
Why should I use functions in Python?
Functions improve code reuse, organization, and readability.
How do I handle errors in Python?
Use try-except blocks to catch and manage exceptions.
What are some useful Python libraries for beginners?
Pandas (data handling), NumPy (math), Requests (web), and Tkinter (GUI).
What is the difference between for and while loops?
for loops iterate over sequences, while while loops run until a condition is met.
Can I automate tasks with Python?
Yes! Python is great for automating repetitive tasks using scripts.
How can I improve my Python coding skills?
Practice regularly, read documentation, and contribute to open-source projects.
What are the career opportunities with Python?
Python is used in web development, data science, AI, cybersecurity, and automation.
The Python Full Stack Training is a 2 months program offering online and offline classes. We covers Python fundamentals, front-end technologies like HTML training, CSS training, and React training and back-end frameworks such as Django and Flask. With hands-on project , expert guidance and free batch access until get placement."