Python Full Stack Developer Skills
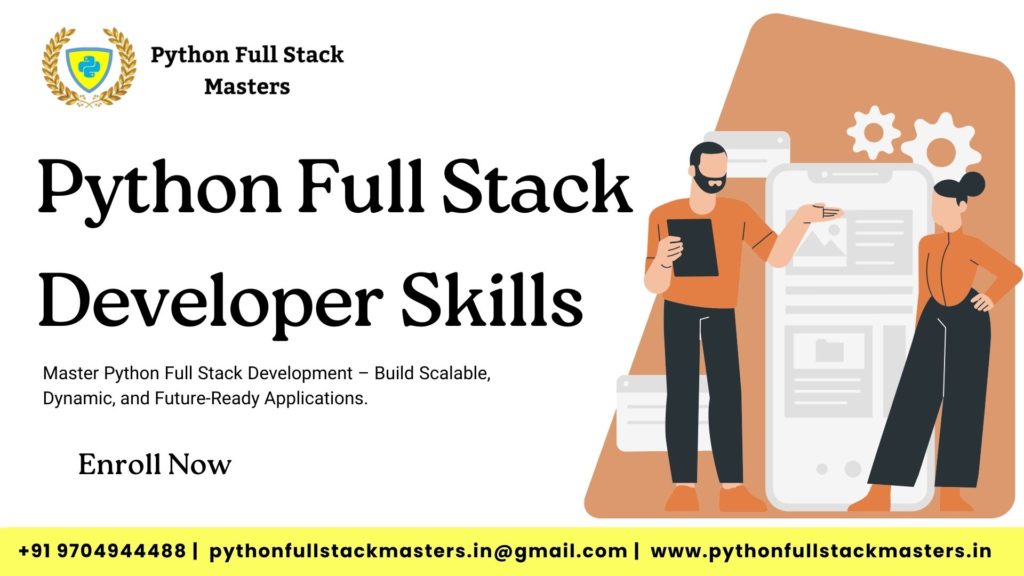
Introduction
1. Who is a Python Full Stack Developer?
- A Python Full Stack Developer Skills is a software professional skilled in both frontend and backend development, utilizing Python as the core backend technology. These developers can build complete web applications, manage databases, optimize performance, and deploy scalable applications.
Key responsibilities of a Python Full Stack Developer Skills include:
- Designing interactive user interfaces using HTML, CSS, and JavaScript.
- Developing backend logic with Python frameworks like Django or Flask.
- Managing databases (SQL and NoSQL) for efficient data storage and retrieval.
- Ensuring application security and performance optimization.
- Deploying applications using Docker, Kubernetes, or cloud platforms.
- Python Full Stack Developers are in high demand due to their versatility and ability to handle end-to-end development, making them an invaluable asset to any tech team.
2. Why Python for Full Stack Development Skills ?
- Python Full Stack Developer Skills
- Python is one of the most popular programming languages for full stack development, and for good reasons:
- Simplicity and Readability
- Python’s syntax is clean and beginner-friendly, making it easier for developers to learn and write maintainable code.
- Rich Ecosystem of Libraries and Frameworks
- Python provides powerful frameworks like Django and Flask for backend development, along with vast libraries for data processing, AI, and more.
- Scalability and Flexibility
- Python applications can be scaled efficiently, and the language is widely used in various fields, including web development, AI, automation, and data science.
- Strong Community Support
- With millions of active developers, Python has extensive documentation, tutorials, and support forums.
- Integration with Modern Technologies
- Python can seamlessly integrate with machine learning, IoT, and cloud services, making it future-proof.
Frontend Skills
3. HTML, CSS, and JavaScript Mastery
- Frontend development is crucial for creating an engaging user experience. A Python Full Stack Developer must master the following:
- HTML (HyperText Markup Language)
- Defines the structure of web pages.
- Uses elements like <div>, <span>, <h1> – <h6>, <p>, and <img>.
- Forms the foundation of web development.
- CSS (Cascading Style Sheets)
- Styles web pages for better visual appeal.
- CSS properties include color, font-size, margin, and padding.
- Advanced techniques like CSS Grid and Flexbox ensure responsive layouts.
- JavaScript (JS)
- Adds interactivity to websites.
- ES6+ features like Arrow functions, Async/Await, and Promises improve functionality.
- Used in frontend frameworks like React and Vue.js.
- Mastering these three technologies ensures a Python Full Stack Developer can build responsive, interactive, and user-friendly applications.
4. Frontend Frameworks (React, Vue, Angular)
- While HTML, CSS, and JavaScript form the foundation of frontend development, modern applications require frontend frameworks to build efficient and scalable UIs.
- React.js Developed by Facebook.
- Uses a component-based architecture for reusability.
- Implements Virtual DOM for faster rendering.
- Vue.js
- Lightweight and beginner-friendly.
- Uses a reactive data-binding system.
- Ideal for small to medium projects.
- Angular
- Developed by Google.
- Uses TypeScript for better maintainability.
- Best suited for large-scale enterprise applications.
- Each framework has its strengths, but React.js is the most popular choice due to its performance and flexibility.
5. Responsive Design and UI Optimization
- A well-designed web application must be responsive and optimized for different devices.
- Key Concepts in Responsive Design:
- Media Queries: Adapting layouts based on screen size (@media in CSS).
- CSS Flexbox and Grid: Creating dynamic, flexible layouts.
- Mobile-First Design: Ensuring the website works on mobile devices before scaling to larger screens.
- Bootstrap & Tailwind CSS: Pre-built UI libraries for faster development.
- UI Performance Optimization:
- Lazy Loading Images: Loading images only when needed to improve page speed.
- Minifying CSS and JavaScript: Reducing file sizes for faster loading.
- Using a Content Delivery Network (CDN): Serving assets from a global network for faster access.
Backend Skills
6. Python Fundamentals and Advanced Concepts
- A Python Full Stack Developer must have a strong grasp of Python fundamentals before diving into backend frameworks. Some key concepts include:
- Basic Python Syntax
- Variables, Data Types (int, float, string, list, tuple, dict, set).
- Conditional Statements (if, elif, else).
- Loops (for, while).
- Functions and Object-Oriented Programming (OOP)
- Writing reusable functions using def.
- Understanding Classes and Objects for modular programming.
- OOP principles: Encapsulation, Abstraction, Inheritance, and Polymorphism.
- Error Handling and Debugging
- Using try-except-finally for exception handling.
- Debugging with tools like PDB (Python Debugger).
- Advanced Python Concepts
- Decorators: Enhancing function behavior.
- Generators and Iterators: Memory-efficient looping.
- Concurrency & Multithreading: Using asyncio and threading for performance optimization.
- A strong foundation in Python ensures developers can efficiently build and scale backend applications.
7. Django and Flask: Python Backend Frameworks
- Python provides powerful frameworks for backend development. The two most popular are:
- Django (High-Level Framework)
- Django follows the MTV (Model-Template-View) architecture, making it an excellent choice for rapid development.
Why Choose Django?
- Batteries-Included Approach: Comes with built-in authentication, ORM, and admin panel.
- Security: Protects against SQL injection, XSS, and CSRF attacks.
- Scalability: Used by Instagram, Pinterest, and Disqus.
- Flask (Lightweight Microframework)
- Flask is minimalistic and gives developers more flexibility to choose their stack.
Why Choose Flask?
- Lightweight: No built-in features, giving more control.
- Easier Learning Curve: Ideal for small projects and APIs.
- Used by Netflix and Reddit: Proving its production readiness.
- Both frameworks have their advantages:
- Use Django for large-scale applications.
- Use Flask for small, lightweight apps and microservices.
8. API Development (RESTful and GraphQL)
- A backend must communicate efficiently with the frontend using APIs (Application Programming Interfaces). There are two main API standards:
- RESTful APIs (Representational State Transfer)
- Uses HTTP methods: GET, POST, PUT, DELETE.
- Uses JSON to exchange data.
- Example: https://api.example.com/users returns all users.
- Tools for RESTful API Development in Python:
- Django REST Framework (DRF) for Django applications.
- Flask-RESTful for Flask applications.
- GraphQL APIs
- Reduces over-fetching and under-fetching of data.
- Used by Facebook, GitHub, and Shopify.
- A Python Full Stack Developer should understand both REST and GraphQL to build efficient, scalable APIs.
Database Management
9. SQL and NoSQL Database Mastery
- Databases store and manage application data. Developers must choose the right database based on project requirements.
- SQL Databases (Relational)
- Popular SQL databases include:
- PostgreSQL: Open-source, highly scalable, and widely used.
- MySQL: Popular and easy to set up.
- SQLite: Lightweight database used for local applications.
Advantages of SQL:
ACID compliance ensures data integrity.
Ideal for applications requiring structured data.
- NoSQL Databases (Non-Relational)
- NoSQL databases store unstructured or semi-structured data and are used in high-performance applications. Examples include:
- MongoDB: Stores data in JSON-like documents.
- Firebase: A real-time NoSQL database from Google.
Advantages of NoSQL:
Faster performance for big data applications.
Scales easily across multiple servers.
- A Python Full Stack Developer should understand when to use SQL vs. NoSQL based on project needs.
10. ORM (Object-Relational Mapping) and Query Optimization
- Instead of writing raw SQL queries, developers can use ORMs to interact with databases using Python code.
- Popular ORMs for Python:
- Django ORM (for Django projects).
- SQLAlchemy (for Flask and general Python use).
Benefits of ORMs:
Prevents SQL injection attacks.
Increases development speed.
Makes database queries easier to read and maintain.
- Query Optimization Techniques:
- Indexing: Improves query performance.
- Caching: Storing frequent queries in memory.
- Avoiding N+1 Queries: Optimizing database relationships.
- Using ORMs with optimized queries ensures a database performs efficiently.
Version Control and Deployment
11. Git, GitHub, and Best Version Control Practices
- Python Full Stack Developers should master:
- Git Basics:
- git init → Initialize a repository.
- git add . → Stage changes.
- git commit -m “message” → Save changes.
- git push origin main → Push changes to GitHub.
- Branching and Merging:
- Using git branch feature-branch for new features.
- git merge feature-branch to merge changes.
- Resolving merge conflicts efficiently.
- Using GitHub and GitLab:
- Pull Requests: Reviewing and merging code.
- Issue Tracking: Managing tasks and bug reports.
- Mastering Git and GitHub helps developers collaborate seamlessly in teams.
12. CI/CD Pipelines for Automated Deployment
- Continuous Integration and Continuous Deployment (CI/CD) automate testing and deployment.
CI/CD Workflow:
1.Code Commit → Developer pushes code to GitHub.
2. Automated Tests → Tools like Jenkins or GitHub Actions run tests.
3. Build & Deploy → Successful builds are deployed automatically.
Popular CI/CD Tools:
- Jenkins → Open-source automation tool.
- GitHub Actions → Automates workflows directly in GitHub.
- Travis CI & CircleCI → Cloud-based CI/CD solutions.
- CI/CD improves efficiency and reduces deployment errors.
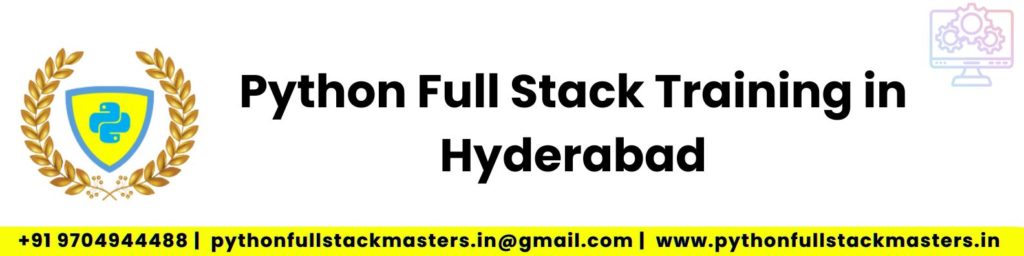
13. Docker, Kubernetes, and Containerization
- Containerization allows developers to package applications with all dependencies, ensuring they run consistently across different environments.
- Docker: Creates lightweight, portable containers.
- Dockerfile defines how an app is built and runs.
- Uses docker-compose to manage multi-container applications.
- Kubernetes:
- Orchestrates containers in large-scale applications.
- Ensures load balancing, auto-scaling, and failover management.
- Used by Google, Spotify, and Airbnb.
Why Use Containers?
- Eliminates “Works on my machine” issues.
- Scales applications effortlessly.
Security and Performance Optimization
14. Web Security Best Practices for Full Stack Developers
- A Python Full Stack Developer must implement strong security measures to protect applications from cyber threats.
- Preventing SQL Injection
- SQL injection occurs when malicious users manipulate input fields to execute harmful SQL queries. To prevent this:
- Use parameterized queries instead of raw SQL.
- Utilize ORMs like Django ORM or SQLAlchemy, which automatically prevent injections.
- Preventing Cross-Site Scripting (XSS)
- XSS attacks inject malicious scripts into web pages. Prevention techniques include:
- Sanitizing user inputs with libraries like Bleach (Python).
- Implementing Content Security Policy (CSP) headers.
- Cross-Site Request Forgery (CSRF) Protection
- Attackers can exploit authenticated users to perform unintended actions. Prevent CSRF by:
- Using CSRF tokens in forms and API requests.
- Leveraging Django’s built-in CSRF protection.
- Implementing Secure Authentication & Authorization
- Use OAuth 2.0, JWT (JSON Web Tokens), or OpenID Connect for secure authentication.
- Hash passwords using bcrypt or Argon2 instead of storing them in plain text.
- Implement role-based access control (RBAC) to limit permissions.
- A secure application builds user trust and protects sensitive data from cyber threats.
15. Performance Optimization and Scalability
- A slow web application drives users away. A Python Full Stack Developer must optimize applications for performance and scalability.
- Optimizing Database Performance
- Use indexes to speed up queries.
- Optimize queries by avoiding unnecessary joins and selecting only required columns.
- Implement caching mechanisms (Redis, Memcached) to reduce database load.
- Improving Backend Performance
- Use asynchronous programming with asyncio and Celery to handle background tasks.
- Optimize API performance using pagination and request throttling.
- Implement load balancing to distribute requests efficiently.
- Frontend Optimization Techniques
- Minify CSS, JavaScript, and images to reduce load time.
- Implement lazy loading for images and heavy resources.
- Scalability ensures applications handle increasing user loads efficiently without performance drops.
Soft Skills and Collaboration
16. Problem-Solving and Debugging Skills
- Technical skills alone don’t make a great developer. Strong problem-solving and debugging skills help developers:
- Identify performance bottlenecks.
Fix broken features.
- Optimize existing code for efficiency.
- Debugging Techniques
- Use Python’s logging module for better insights.
- Utilize breakpoints and debugging tools like PDB and VS Code Debugger.
- Test code with unit tests (PyTest, Unittest) before deployment.
- Algorithmic Thinking
- Knowledge of data structures (lists, dictionaries, sets, trees) improves efficiency.
- Implement searching and sorting algorithms for faster data processing.
- Use Big-O analysis to evaluate algorithm performance.
- A Python Full Stack Developer must approach problem-solving logically and systematically.
17. Team Collaboration and Agile Methodology
- Developers rarely work alone; they collaborate with UI/UX designers, DevOps engineers, and product managers.
- Agile Development and Scrum
- Agile methodology follows incremental development cycles.
- Developers work in sprints (2-week cycles) with specific goals.
- Regular stand-up meetings ensure alignment between team members.
- Effective Communication
- Use tools like Slack, Microsoft Teams, or Discord for instant collaboration.
- Write clear documentation for APIs and codebases.
- Participate in code reviews to maintain quality standards.
- Strong communication and teamwork ensure projects are delivered on time and with high quality.
Advanced Technologies for Python Full Stack Developers
18. Cloud Computing (AWS, Google Cloud, Azure)
- Cloud platforms enable developers to deploy and manage applications efficiently.
- Key Cloud Services
- Compute Services: AWS EC2, Google Compute Engine.
- Storage Solutions: AWS S3, Google Cloud Storage.
- Database Services: AWS RDS, Firebase, MongoDB Atlas.
- Serverless Computing: AWS Lambda, Google Cloud Functions.
- Cloud Deployment Best Practices
- Automate deployment using CI/CD pipelines.
- Use Infrastructure as Code (IaC) tools like Terraform.
- Implement cloud security best practices (IAM roles, encryption).
- Cloud computing skills are essential for modern web application development.
19. AI and Machine Learning Integration in Full Stack Development
- Python is a leading language for AI and ML, and full stack developers can integrate AI-driven features into their applications.
- AI-Powered Features in Web Applications
- Chatbots & Virtual Assistants using NLP libraries like spaCy.
- Recommendation Systems using ML models trained with Scikit-learn.
- Image Recognition with TensorFlow and OpenCV.
- Deploying AI Models in Web Apps
- Convert ML models into APIs using Flask or FastAPI.
- Use TensorFlow.js to run ML models in the browser.
- Optimize AI models for low latency and high scalability.
- AI integration enhances user experience by providing personalized and intelligent features.
20. Future Trends in Python Full Stack Development
- Technology evolves constantly. Python Full Stack Developers must stay updated with the latest trends to remain competitive.
- Web 3.0 and Blockchain Development
- Decentralized apps (DApps) using Web3.py.
- Smart contracts on Ethereum and Solana.
- Server less and Edge Computing
- Reducing backend infrastructure using AWS Lambda, Google Cloud Run.
- Processing data closer to users via edge computing.
- AI-Powered Web Development
- AI-driven tools like GitHub Copilot for code suggestions.
- AI-generated content and automation.
- The future of full stack development is exciting and filled with opportunities for those who stay ahead of the curve.
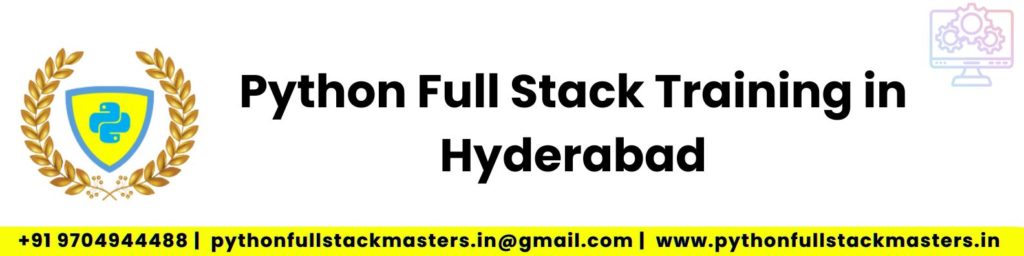
Conclusion
Becoming a Python Full Stack Developer requires mastering a diverse set of technical and soft skills. From frontend development to backend architecture, database management, and cloud computing, a skilled developer can build and deploy high-performance applications efficiently.
To stay relevant in the industry:
Continuously learn new technologies.
Work on real-world projects to gain practical experience.
Contribute to open-source projects and participate in coding communities.
By developing a strong foundation in Python and full stack technologies, you’ll open doors to high-paying job opportunities and exciting career growth in the software development industry.
FAQs
1. How long does it take to become a Python Full Stack Developer?
Beginners need 6-12 months of consistent learning and project-building to become proficient.
2. Is Python enough for full stack development?
Python is great for backend (Django, Flask), but you also need HTML, CSS, JavaScript, and frontend frameworks (React, Vue).
3. What is the average salary of a Python Full Stack Developer?
In the U.S., salaries range from $90,000 to $140,000/year, depending on experience.
4. What projects can a Python Full Stack Developer work on?
E-commerce, SaaS apps, chat apps, AI-powered platforms, real-time dashboards, and more.
5. Can I learn full stack development without a CS degree?
Yes! Many self-taught developers use online courses, coding bootcamps, and practice projects to get hired.
6. What are some beginner-friendly full stack projects?
Try To-Do List App, Blog Platform, Weather App, E-Commerce Website, or Chat Application to build experience.
7. Is Python better than JavaScript for full stack development?
Python is great for backend and AI/ML, while JavaScript (Node.js) is better for real-time applications.
8. What databases should a Python Full Stack Developer know?
SQL: PostgreSQL, MySQL, SQLite.
NoSQL: MongoDB, Firebase.
9. What DevOps skills are useful for Python Full Stack Developers?
Learn Git, Docker, Kubernetes, CI/CD, AWS, and Terraform for deployment and scalability.
10. What are the latest trends in Python Full Stack Development?
Web 3.0, AI integration, Serverless Computing, Edge Computing, and Microservices Architecture.
The Python Full Stack Training is a 2 months program offering online and offline classes. We covers Python fundamentals, front-end technologies like HTML training, CSS training, and React training and back-end frameworks such as Django and Flask. With hands-on project , expert guidance and free batch access until get placement."