Table of Contents
TogglePython Questions For Practice
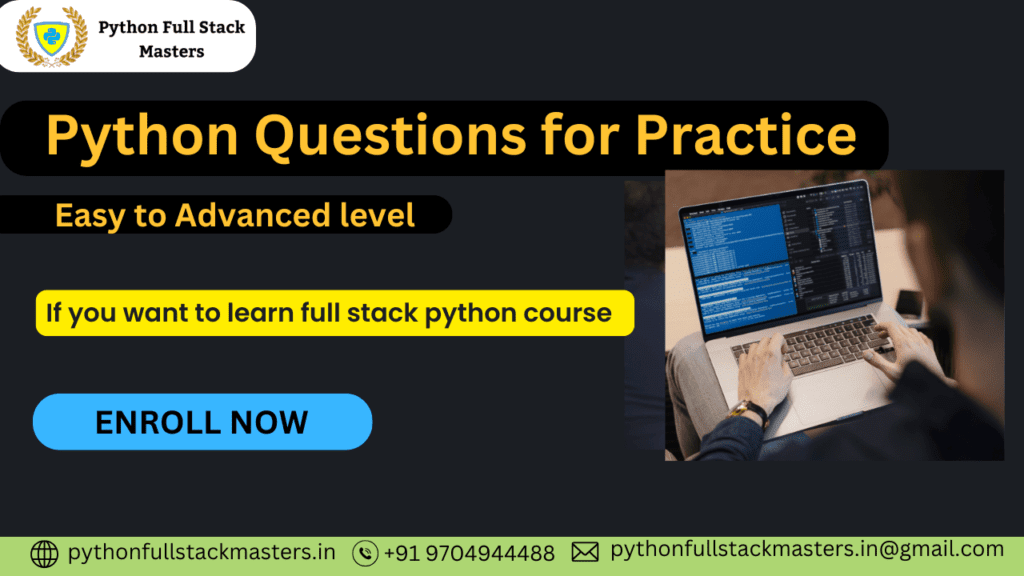
- Are you looking for the best Python practice questions to improve your coding skills? Whether you’re a beginner starting out or an advanced programmer looking to refine your expertise, practicing Python problems is the key to mastering the language.
- In this blog, we have curated 50+ Python coding questions, categorized into Easy, Intermediate, and Advanced levels, to help you strengthen your problem-solving skills. From basic syntax to complex algorithms, these exercises cover a wide range of topics, including:
- String manipulation
- Data structures (lists, dictionaries, sets)
- Algorithms (sorting, searching, recursion)
- Object-oriented programming
- Real-world applications
- Each question comes with a clear explanation and solution, ensuring you not only write code but also understand the logic behind it.
- These problems are perfect for Python learners, coding interview preparation, competitive programming, and technical job assessments.
Why to practice these Python questions?
Enhance your coding skills and logical thinking
Prepare for technical interviews and coding challenges
Build confidence in writing efficient and optimized Python code
Top 20 Easy Level Python Questions For Practice with code
1. Print "Hello, World!"
Concept: The print() function is used to display output in Python.
Code:
print(“Hello, World!”)
Explanation:
- print() is a built-in function that outputs text to the console.
- The text inside quotes (“Hello, World!”) is printed exactly as it appears.
2. Swap two numbers without using a third variable
Concept: Python allows multiple assignments in a single line.
Code:
a, b = 5, 10
a, b = b, a
print(a, b)
Explanation:
- The values of a and b are swapped directly using tuple unpacking.
- This eliminates the need for a temporary variable.
3. Check if a number is even or odd
Concept: The modulo operator % is used to check divisibility.
Code:
num = int(input(“Enter a number: “))
if num % 2 == 0:
print(“Even”)
else:
print(“Odd”)
Explanation:
- If a number is divisible by 2 (num % 2 == 0), it is even; otherwise, it is odd.
- input() takes user input, which is converted to an integer using int().
4. Find the largest number among three numbers
Concept: The max() function or conditional statements can be used.
Code:
a, b, c = map(int, input(“Enter three numbers: “).split())
print(“Largest number is:”, max(a, b, c))
Explanation:
- max(a, b, c) returns the maximum of the three numbers.
- map(int, input().split()) allows multiple inputs in a single line.
5. Calculate the sum of the first N natural numbers
Concept: The sum of the first N natural numbers is given by the formula N * (N + 1) / 2.
Code:
n = int(input(“Enter N: “))
print(“Sum:”, n * (n + 1) // 2)
Explanation:
- The formula efficiently calculates the sum in O(1) time complexity.
- // is used for integer division.
6. Find the factorial of a number
Concept: Factorial of n (denoted as n!) is the product of all integers from 1 to n.
Code:
def factorial(n):
return 1 if n == 0 else n * factorial(n – 1)
num = int(input(“Enter a number: “))
print(“Factorial:”, factorial(num))
Explanation:
- This is a recursive function, meaning it calls itself with a smaller value until n == 0.
The base case (n == 0) returns 1 to stop recursion
7. Check if a number is a prime number
Concept: A prime number has only two divisors: 1 and itself.
Code:
num = int(input(“Enter a number: “))
if num > 1:
for i in range(2, int(num ** 0.5) + 1):
if num % i == 0:
print(“Not Prime”)
break
else:
print(“Prime”)
else:
print(“Not Prime”)
Explanation:
- We check divisibility from 2 to sqrt(num), which reduces time complexity.
- If num has any divisor other than 1 and itself, it is not prime.
8. Print the Fibonacci series up to N terms
Concept: Fibonacci sequence starts with 0, 1, and each subsequent number is the sum of the previous two.
Code:
n = int(input(“Enter N: “))
a, b = 0, 1
for _ in range(n):
print(a, end=” “)
a, b = b, a + b
Explanation:
- We initialize a=0 and b=1, then generate the series iteratively.
- The loop prints the series up to N terms.
9. Reverse a string
Concept: Strings can be reversed using slicing.
Code:
s = input(“Enter a string: “)
print(“Reversed:”, s[::-1])
Explanation:
- [::-1] creates a reversed copy of the string using slicing.
- This is an efficient way to reverse strings in Python.
10. Check if a string is a palindrome
Concept: A palindrome reads the same forward and backward.
Code:
s = input(“Enter a string: “)
print(“Palindrome” if s == s[::-1] else “Not Palindrome”)
Explanation:
- The string is reversed using slicing (s[::-1]) and compared with the original.
- If both are equal, the string is a palindrome.
11. Find the sum of the digits of a number
Explanation:
To find the sum of the digits of a number, we extract each digit using modulus (%) and division (//) operations or convert the number to a string and sum up the digits.
Code:
num = int(input(“Enter a number: “))
sum_digits = sum(int(digit) for digit in str(num))
print(“Sum of digits:”, sum_digits)
Logic: We convert the number to a string and sum up each digit by converting it back to an integer.
12. Check if a year is a leap year
Explanation:
A leap year is divisible by 4, but if it’s a century year (divisible by 100), it should also be divisible by 400.
Code:
year = int(input(“Enter a year: “))
if (year % 4 == 0 and year % 100 != 0) or (year % 400 == 0):
print(year, “is a Leap Year”)
else:
print(year, “is not a Leap Year”)
Logic:
- If a year is divisible by 400, it’s a leap year.
- If it’s divisible by 4 but not by 100, it’s a leap year.
- Otherwise, it’s not a leap year.
13. Count the number of vowels in a string
Explanation:
We check each character in a string and count how many of them are vowels (a, e, i, o, u in both lowercase and uppercase).
Code:
s = input(“Enter a string: “).lower()
vowel_count = sum(1 for ch in s if ch in “aeiou”)
print(“Number of vowels:”, vowel_count)
Logic:
- Convert the string to lowercase to handle both uppercase and lowercase vowels.
- Loop through each character and count how many are vowels.
14. Find the greatest common divisor (GCD) of two numbers
Explanation:
The GCD of two numbers is the largest number that divides both numbers completely. We can use Python’s built-in math.gcd() function.
Code:
import math
a, b = map(int, input(“Enter two numbers: “).split())
print(“GCD:”, math.gcd(a, b))
Logic:
- We use math.gcd(a, b) to find the greatest common divisor of a and b.
15. Reverse a number
Explanation:
To reverse a number, we can convert it into a string and reverse the string representation.
Code:
num = int(input(“Enter a number: “))
reversed_num = int(str(num)[::-1])
print(“Reversed Number:”, reversed_num)
Logic: Convert the number into a string, reverse it using slicing ([::-1]), and convert it back to an integer.
16. Find the ASCII value of a character
Explanation:
ASCII (American Standard Code for Information Interchange) assigns a numerical value to each character. We use ord() to get the ASCII value.
Code:
ch = input(“Enter a character: “)
print(“ASCII value of”, ch, “is”, ord(ch))
Logic: The ord() function returns the ASCII value of a character.
17. Check if a number is an Armstrong number
Explanation:
An Armstrong number (also called a narcissistic number) is a number where the sum of its digits raised to the power of the number of digits equals the number itself.
For example, 153 is an Armstrong number because:
13+53+33=1531^3 + 5^3 + 3^3 = 15313+53+33=153
Code:
num = int(input(“Enter a number: “))
order = len(str(num))
armstrong_sum = sum(int(digit) ** order for digit in str(num))
if num == armstrong_sum:
print(num, “is an Armstrong number”)
else:
print(num, “is not an Armstrong number”)
Logic:
- Calculate the number of digits (order).
- Raise each digit to the power of order and sum the results.
- If the sum matches the original number, it’s an Armstrong number.
18. Find the least common multiple (LCM) of two numbers
Explanation:
The LCM is the smallest number that is a multiple of both numbers. We can calculate it using the formula:
LCM(a,b)=∣a×b∣GCD(a,b)LCM(a, b) = \frac{|a \times b|}{GCD(a, b)}LCM(a,b)=GCD(a,b)∣a×b∣
Python provides a built-in function to find the LCM.
Code:
import math
a, b = map(int, input(“Enter two numbers: “).split())
print(“LCM:”, math.lcm(a, b))
Logic:
- math.lcm(a, b) calculates the least common multiple.
19. Find the length of a list without using len()
Explanation:
To find the length of a list without len(), we can iterate through the list and count the elements manually.
Code:
lst = [10, 20, 30, 40, 50]
count = sum(1 for _ in lst)
print(“Length of the list:”, count)
Logic: We loop through the list and count the number of elements.
20. Remove duplicates from a list
Explanation:
A list may contain duplicate values. We can remove them using set() since sets do not allow duplicates.
Code:
lst = [1, 2, 2, 3, 4, 4, 5]
unique_lst = list(set(lst))
print(“List after removing duplicates:”, unique_lst)
Logic: Convert the list into a set to remove duplicates and convert it back to a list.
Top 20 Intermediate Level python practice questions
1. Sort a list without using the sort() function
Explanation:
Sorting is the process of arranging elements in ascending or descending order. One way to sort a list without using the built-in sort() function is by implementing the Bubble Sort algorithm, which repeatedly swaps adjacent elements if they are in the wrong order.
Code:
def bubble_sort(lst):
n = len(lst)
for i in range(n):
for j in range(0, n – i – 1):
if lst[j] > lst[j + 1]:
lst[j], lst[j + 1] = lst[j + 1], lst[j] # Swap elements
return lst
numbers = [5, 3, 8, 1, 2]
print(bubble_sort(numbers))
2. Find the second largest number in a list
Explanation:
To find the second largest number, we need to first determine the largest number and then find the next highest value. Using set() helps remove duplicates, and sorting simplifies the selection.
Code:
def second_largest(lst):
unique_numbers = list(set(lst)) # Remove duplicates
unique_numbers.sort(reverse=True) # Sort in descending order
return unique_numbers[1] if len(unique_numbers) > 1 else None # Return second largest
numbers = [10, 20, 4, 45, 99, 99]
print(second_largest(numbers))
3. Check if two strings are anagrams
Explanation:
Two words are anagrams if they contain the same characters in a different order (e.g., “listen” and “silent”). A simple way to check this is by sorting both strings and comparing them.
Code:
def are_anagrams(str1, str2):
return sorted(str1) == sorted(str2)
print(are_anagrams(“listen”, “silent”)) # True
print(are_anagrams(“hello”, “world”)) # False
4. Find common elements between two lists
Explanation:
The common elements between two lists can be found using set operations (& for intersection).
Code:
def common_elements(lst1, lst2):
return list(set(lst1) & set(lst2))
list1 = [1, 2, 3, 4, 5]
list2 = [3, 4, 5, 6, 7]
print(common_elements(list1, list2)) # Output: [3, 4, 5]
5. Find the intersection of two sets
Explanation:
The intersection of two sets contains only the elements that are present in both sets. We use the & operator or the intersection() method.
Code:
set1 = {1, 2, 3, 4, 5}
set2 = {3, 4, 5, 6, 7}
intersection = set1 & set2 # Using & operator
print(intersection) # Output: {3, 4, 5}
# Alternative:
print(set1.intersection(set2)) # Output: {3, 4, 5}
6. Find the union of two sets
Explanation:
The union of two sets contains all unique elements from both sets. We use the | operator or the union() method.
Code:
set1 = {1, 2, 3}
set2 = {3, 4, 5}
union_set = set1 | set2 # Using | operator
print(union_set) # Output: {1, 2, 3, 4, 5}
# Alternative:
print(set1.union(set2)) # Output: {1, 2, 3, 4, 5}
7. Merge two dictionaries
Explanation:
Merging dictionaries means combining their key-value pairs. If the same key appears in both, the second dictionary’s value will overwrite the first one’s.
Code:
dict1 = {‘a’: 1, ‘b’: 2}
dict2 = {‘b’: 3, ‘c’: 4}
# Using dictionary unpacking
merged_dict = {**dict1, **dict2}
print(merged_dict) # Output: {‘a’: 1, ‘b’: 3, ‘c’: 4}
# Alternative using update()
dict1.update(dict2)
print(dict1) # Output: {‘a’: 1, ‘b’: 3, ‘c’: 4}
8. Count occurrences of each word in a string
Explanation:
We split the string into words and use a dictionary to keep track of word frequencies.
Code:
from collections import Counter
def word_count(sentence):
words = sentence.split()
return dict(Counter(words))
text = “hello world hello Python”
print(word_count(text))
# Output: {‘hello’: 2, ‘world’: 1, ‘Python’: 1}
9. Check if a string is a pangram
Explanation:
A pangram is a sentence that contains every letter of the alphabet at least once (e.g., “The quick brown fox jumps over the lazy dog”). We check if the set of letters in the sentence includes all 26 letters.
Code:
import string
def is_pangram(sentence):
return set(string.ascii_lowercase).issubset(set(sentence.lower()))
print(is_pangram(“The quick brown fox jumps over the lazy dog”)) # True
print(is_pangram(“Hello world”)) # False
10. Find the frequency of characters in a string
Explanation:
This problem involves counting how many times each character appears in a string. We can use a dictionary or Counter from the collections module.
Code:
from collections import Counter
def char_frequency(s):
return dict(Counter(s))
text = “hello world”
print(char_frequency(text))
# Output: {‘h’: 1, ‘e’: 1, ‘l’: 3, ‘o’: 2, ‘ ‘: 1, ‘w’: 1, ‘r’: 1, ‘d’: 1}
11. Generate a Random Password of a Given Length
Concept:
A random password typically consists of a mix of uppercase letters, lowercase letters, numbers, and special characters. The random and string modules in Python can help generate a secure password.
Code:
import random
import string
def generate_password(length):
characters = string.ascii_letters + string.digits + string.punctuation
return ”.join(random.choice(characters) for _ in range(length))
print(generate_password(12))
Explanation:
- string.ascii_letters: Contains both uppercase and lowercase letters.
- string.digits: Contains numbers 0-9.
- string.punctuation: Contains special characters.
- random.choice(): Randomly selects characters from the given set.
12. Find the First Non-Repeating Character in a String
Concept:
A non-repeating character appears only once in a string. We can use the collections.Counter module to count occurrences and then find the first character with a count of 1.
Code:
from collections import Counter
def first_non_repeating(s):
count = Counter(s)
for ch in s:
if count[ch] == 1:
return ch
return None
print(first_non_repeating(“swiss”))
Explanation:
- Counter(s): Counts occurrences of each character.
- Iterates through the string and finds the first character with a count of 1.
13. Find the Longest Word in a String
Concept:
To find the longest word, we split the string into words and determine the maximum-length word using the max() function.
Code:
def longest_word(sentence):
words = sentence.split()
return max(words, key=len)
print(longest_word(“Python is a powerful programming language”))
Explanation:
- sentence.split(): Splits the sentence into words.
- max(words, key=len): Finds the longest word based on its length.
14. Reverse Words in a Sentence
Concept:
Reversing words in a sentence means keeping the order of letters in words but changing the sequence of words.
Code:
def reverse_words(sentence):
return ‘ ‘.join(sentence.split()[::-1])
print(reverse_words(“Hello World Python”))
Explanation:
- sentence.split(): Splits the sentence into words.
- [::-1]: Reverses the list of words.
- ‘ ‘.join(): Joins the words back into a sentence.
15. Convert a List of Tuples into a Dictionary
Concept:
A dictionary in Python maps keys to values. If we have a list of tuples where each tuple contains a key-value pair, we can convert it into a dictionary using dict().
Code:
def convert_to_dict(tuples):
return dict(tuples)
tuples_list = [(“name”, “Alice”), (“age”, 25), (“city”, “New York”)]
print(convert_to_dict(tuples_list))
Explanation:
- dict(tuples): Converts a list of tuples into a dictionary.
16. Find All Unique Elements in a List
Concept:
A set in Python stores only unique values. Converting a list to a set removes duplicates.
Code:
def unique_elements(lst):
return list(set(lst))
print(unique_elements([1, 2, 2, 3, 4, 4, 5]))
Explanation:
- set(lst): Removes duplicate elements.
list(set(lst)): Converts the set back into a list.
17. Remove All Occurrences of a Specific Element from a List
Concept:
To remove all occurrences of a given element, we can use list comprehension to filter out that element.
Code:
def remove_element(lst, element):
return [x for x in lst if x != element]
print(remove_element([1, 2, 3, 2, 4, 2, 5], 2))
Explanation:
- List comprehension creates a new list without the unwanted element.
18. Convert a String into Title Case
Concept:
Title case means the first letter of each word is capitalized. Python provides a built-in method .title() for this.
Code:
def to_title_case(sentence):
return sentence.title()
print(to_title_case(“hello world, welcome to python”))
Explanation:
- .title(): Converts the first letter of each word to uppercase.
19. Find the Sum of All Elements in a Nested List
Concept:
A nested list contains lists within a list. To find the sum, we need to flatten the list and add all elements.
Code:
def sum_nested_list(lst):
total = 0
for sublist in lst:
total += sum(sublist)
return total
print(sum_nested_list([[1, 2, 3], [4, 5], [6, 7, 8]]))
Explanation:
- Loops through each sublist and adds its sum to the total.
20. Write a Function to Return the Nth Fibonacci Number
Concept:
The Fibonacci sequence starts with 0 and 1, and each next number is the sum of the previous two numbers.
Code:
def fibonacci(n):
if n <= 0:
return “Invalid Input”
elif n == 1:
return 0
elif n == 2:
return 1
else:
return fibonacci(n – 1) + fibonacci(n – 2)
print(fibonacci(7))
Explanation:
- Uses recursion to calculate the Fibonacci sequence.
fibonacci(n-1) + fibonacci(n-2): Computes the nth Fibonacci number.
Top 10+ Advanced Level python practice questions
1. Generate a Random Password of a Given Length
Concept:
A secure password should be a mix of uppercase, lowercase, numbers, and special characters. Python’s random and string modules help generate random passwords.
Code:
import random
import string
def generate_password(length):
characters = string.ascii_letters + string.digits + string.punctuation
return ”.join(random.choice(characters) for _ in range(length))
print(generate_password(12))
Explanation:
- string.ascii_letters provides uppercase and lowercase letters.
- string.digits includes numbers (0-9).
- string.punctuation adds special characters.
random.choice() selects random characters for the password.
2. Find the First Non-Repeating Character in a String
Concept:
A non-repeating character is one that appears only once in a string. Using collections.Counter, we can count occurrences efficiently.
Code:
from collections import Counter
def first_non_repeating(s):
counts = Counter(s)
for char in s:
if counts[char] == 1:
return char
return None
print(first_non_repeating(“swiss”))
Explanation:
- Counter(s) counts occurrences of each character.
The first character with a count of 1 is returned.
3. Implement a Simple Calculator Using Functions
Concept:
A basic calculator should support operations like addition, subtraction, multiplication, and division.
Code:
def calculator(a, b, operation):
if operation == ‘+’:
return a + b
elif operation == ‘-‘:
return a – b
elif operation == ‘*’:
return a * b
elif operation == ‘/’:
return a / b if b != 0 else “Cannot divide by zero”
else:
return “Invalid operation”
print(calculator(10, 5, ‘+’))
Explanation:
- Uses an if-elif structure to check the operation type.
- Prevents division by zero error.
4. Find All Permutations of a String
Concept:
A permutation is a rearrangement of characters in a string. Python’s itertools.permutations helps generate all possible permutations.
Code:
from itertools import permutations
def string_permutations(s):
return [”.join(p) for p in permutations(s)]
print(string_permutations(“abc”))
Explanation:
- permutations(s) generates all possible arrangements.
- ”.join(p) converts tuples into strings.
5. Implement a Program to Check If a Sudoku Board Is Valid
Concept:
A valid Sudoku board must satisfy these conditions:
- Each row contains numbers 1-9 without repetition.
- Each column contains numbers 1-9 without repetition.
- Each 3×3 sub-grid contains numbers 1-9 without repetition.
Code:
def is_valid_sudoku(board):
def is_valid_unit(unit):
unit = [num for num in unit if num != “.”]
return len(unit) == len(set(unit))
for row in board:
if not is_valid_unit(row):
return False
for col in zip(*board):
if not is_valid_unit(col):
return False
for i in range(0, 9, 3):
for j in range(0, 9, 3):
box = [board[x][y] for x in range(i, i+3) for y in range(j, j+3)]
if not is_valid_unit(box):
return False
return True
Explanation:
- Uses helper function is_valid_unit() to check rows, columns, and sub-grids.
- zip(*board) transposes the board for column checking.
6. Find the Maximum Subarray Sum (Kadane’s Algorithm)
Concept:
Kadane’s algorithm finds the largest sum of a contiguous subarray in O(n) time.
Code:
def max_subarray_sum(arr):
max_sum = current_sum = arr[0]
for num in arr[1:]:
current_sum = max(num, current_sum + num)
max_sum = max(max_sum, current_sum)
return max_sum
print(max_subarray_sum([-2,1,-3,4,-1,2,1,-5,4]))
Explanation:
- Keeps track of the current_sum and updates max_sum.
7. Check If a String Has Balanced Parentheses
Concept:
Balanced parentheses mean each opening ( has a matching closing ).
Code:
def is_balanced(s):
stack = []
for char in s:
if char in “({[“:
stack.append(char)
elif char in “)}]”:
if not stack or “({[“.index(stack.pop()) != “)}]”.index(char):
return False
return not stack
print(is_balanced(“{[()]}”)) # True
Explanation:
- Uses a stack to track matching parentheses.
8. Implement a Basic Contact Book Using a Dictionary
Concept:
A contact book stores names and phone numbers in a dictionary.
Code:
contacts = {}
def add_contact(name, phone):
contacts[name] = phone
def get_contact(name):
return contacts.get(name, “Not found”)
add_contact(“Alice”, “1234567890”)
print(get_contact(“Alice”))
Explanation:
- Uses a dictionary to store contacts.
9. Check If a Number Is a Perfect Number
Concept:
A perfect number is a number whose sum of proper divisors equals itself.
Code:
def is_perfect(n):
return n == sum(i for i in range(1, n) if n % i == 0)
print(is_perfect(28)) # True (28 = 1+2+4+7+14)
Explanation:
- Uses list comprehension to sum divisors.
10. Create a Simple Login System
Concept:
A login system requires a username-password check.
Code:
users = {“user1”: “password123”, “admin”: “admin123”}
def login(username, password):
return “Login Successful” if users.get(username) == password else “Invalid Credentials”
print(login(“user1”, “password123”))
Explanation:
- Uses a dictionary for storing login credentials.
11. Implement a Queue Using a List
Concept:
A queue follows FIFO (First In, First Out) order.
Code:
class Queue:
def __init__(self):
self.queue = []
def enqueue(self, item):
self.queue.append(item)
def dequeue(self):
return self.queue.pop(0) if self.queue else “Queue is empty”
q = Queue()
q.enqueue(1)
q.enqueue(2)
print(q.dequeue()) # 1
Explanation:
- Uses append() for enqueue and pop(0) for dequeue.
12. Find the Shortest Word in a Sentence
Concept:
The shortest word is the one with the least number of characters.
Code:
def shortest_word(sentence):
return min(sentence.split(), key=len)
print(shortest_word(“Python is an amazing language”))
Explanation:
- Uses min() with key=len to find the shortest word.
Additional Resources:
FAQ'S
Can I learn Python in 15 days?
You can learn the basics of Python in 15 days with a structured plan and daily practice. However, it can take months to master Python’s libraries and become proficient.
- Set a plan: Create a detailed plan with time allocations for each day.
- Learn the basics: Learn about Python’s syntax, variables, data types, and operators.
- Practice: Write simple programs to practice your knowledge.
- Build projects: Use datasets and projects from websites like Kaggle or GitHub to build your skills.
What you can learn in 15 days
- How to install Python on your computer
- How to run Python scripts
- How to declare and manipulate variables
- How to perform arithmetic, comparison, and logical operations
- How to make decisions in your Python programs
- How to repeat actions in your programs
Where can I practice Python code?
- Practice Python. If you’re a beginner just starting out with Python, you’ll find Practice Python helpful. …
- Edabit. Edabit is a platform that offers a variety of programming challenges for multiple languages, including Python. …
- CodeWars. …
- Exercism. …
- PYnative. …
- Leetcode. …
- HackerRank.
Can I study Python in 2 days?
In general, it takes around two to six months to learn the fundamentals of Python. But you can learn enough to write your first short program in a matter of minutes. Developing mastery of Python’s vast array of libraries can take months or years.
Is Python basics hard?
What is OOPs in Python?
OOPs concepts in Python) Object Oriented Programming is a way of computer programming using the idea of “objects” to represents data and methods. It is also, an approach used for creating neat and reusable code instead of a redundant one. the program is divided into self-contained objects or several mini-programs.
How to print a pattern in Python?
In Python, we commonly use multiple for loops, and sometimes while loops, too, to print the various patterns. Here, the first outer loop prints the number of rows, and the inner loop prints the number of columns of the pattern.16
What is Python full detail?
How much Python do I need to know to get a job?
The Python Full Stack Training is a 2 months program offering online and offline classes. We covers Python fundamentals, front-end technologies like HTML training, CSS training, and React training and back-end frameworks such as Django and Flask. With hands-on project , expert guidance and free batch access until get placement."